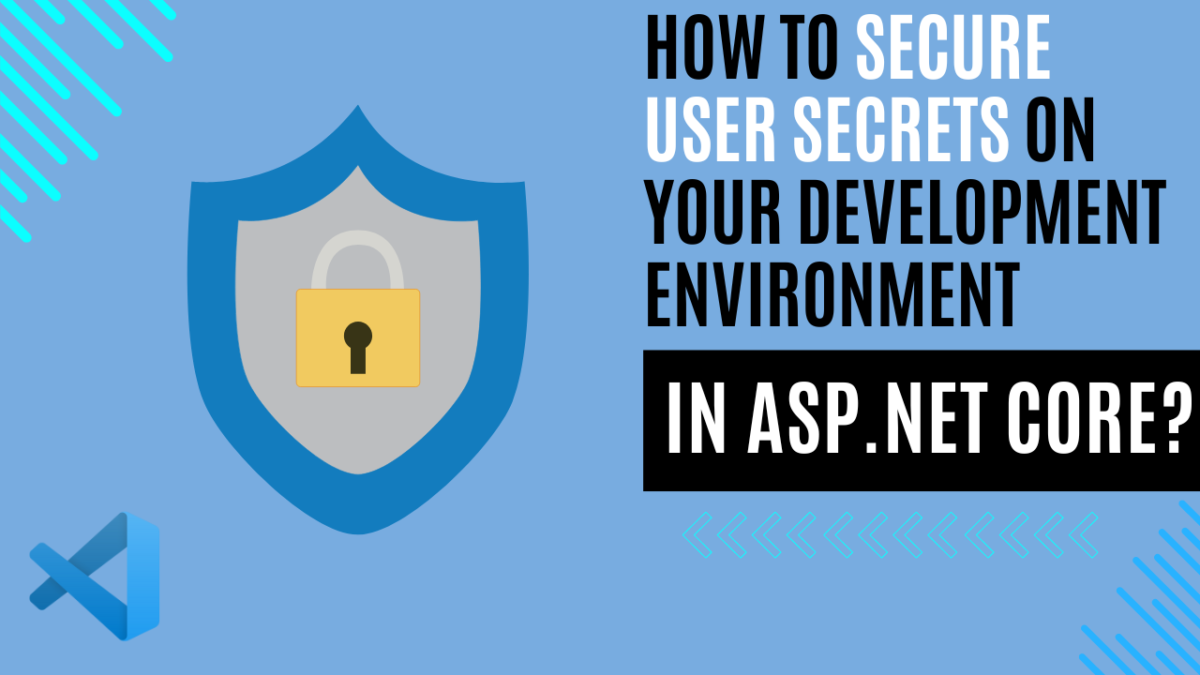
How to Secure User Secrets on Your Development Environment in ASP.NET Core?
Introduction: Safeguarding Sensitive Data in ASP.NET Core Development
When developing applications, we increasingly rely on various services, such as databases and cloud platforms. This reliance necessitates storing connection strings and credentials, which is commonly done in configuration files. However, this approach poses significant risks. Storing sensitive data in configuration files can lead to inadvertent exposure, especially if these files are committed to version control systems like Git. Even in a local development environment, this practice can compromise the security of your application and the integrity of your services.
This article covers how to manage sensitive data for an ASP.NET Core application on a development machine. It is crucial to avoid storing passwords or other sensitive information directly in your source code. Production secrets should never be used for development or testing purposes, nor should they be included when deploying your app. Instead, production secrets should be accessed through controlled methods such as environment variables or Azure Key Vault. Azure Key Vault allows you to securely store and manage your test and production secrets through its configuration provider.
By adhering to these best practices, developers can ensure that sensitive information remains secure and that their applications are protected from potential security breaches.
Using Environment Variables
To avoid storing app secrets in code or local configuration files, environment variables can be used. Environment variables override configuration values for all previously specified configuration sources, providing a more secure way to handle sensitive data.
In an ASP.NET Core web app with Individual User Accounts security, the appsettings.json file includes a default database connection string (DefaultConnection) for LocalDB, which operates without a password in user mode. During deployment, you can replace this value with an environment variable that holds the complete connection string, including sensitive credentials.
It is important to note that environment variables are generally stored in plain, unencrypted text. If the machine or process is compromised, these environment variables can be accessed by untrusted parties. Therefore, additional measures may be required to prevent the disclosure of user secrets.
Secret Manager
The Secret Manager tool is a vital component for securely managing sensitive data during the development of ASP.NET Core projects. App secrets, which encompass sensitive information like API keys or database connection strings, are stored separately from the project directory. This separation ensures that sensitive data remains isolated and is not inadvertently included in source control repositories.
Key Features:
- Secure Storage: App secrets stored using the Secret Manager tool are kept in a secure location outside of the project directory. This practice prevents accidental exposure of sensitive information in version control systems.
- Project Specific: Each ASP.NET Core project can have its own set of app secrets managed by the Secret Manager. This allows for fine-grained control over which secrets are accessible to each project.
- Development Convenience: The Secret Manager tool provides a convenient way to store and retrieve secrets during development without hard-coding them into configuration files or source code.
Usage Considerations:
- Limited Security: It’s important to note that the Secret Manager tool does not encrypt stored secrets. Therefore, it is primarily intended for development purposes rather than production environments where more robust security measures, like Azure Key Vault, are recommended.
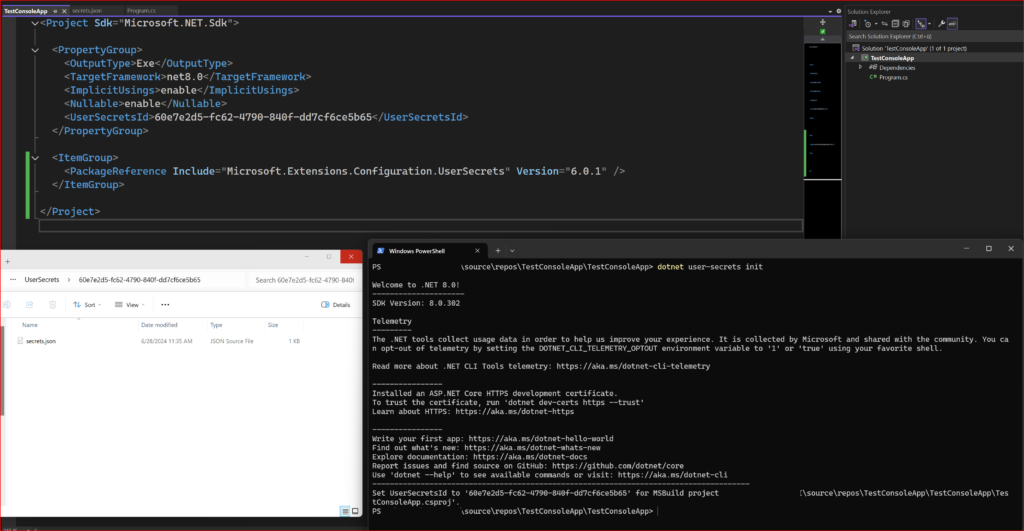
Enable Secret Storage
The Secret Manager tool operates by managing project-specific configuration settings stored in your user profile.
Using the CLI
To utilize user secrets with the Secret Manager tool, execute the following command in your project directory using the .NET CLI:
dotnet user-secrets init
This command adds a UserSecretsId
element within a PropertyGroup
of your project file. By default, the UserSecretsId
is initialized with a GUID. While the GUID itself is arbitrary, it serves as a unique identifier specific to your project.
Using Visual Studio Or Rider
If you prefer using Visual Studio or Rider, you can manage user secrets directly through its interface. Simply right-click on the project in Solution Explorer, then select “Manage User Secrets” from the context menu. This action automatically adds a UserSecretsId
element with a GUID to your project file, enabling you to securely store and manage sensitive data during development.
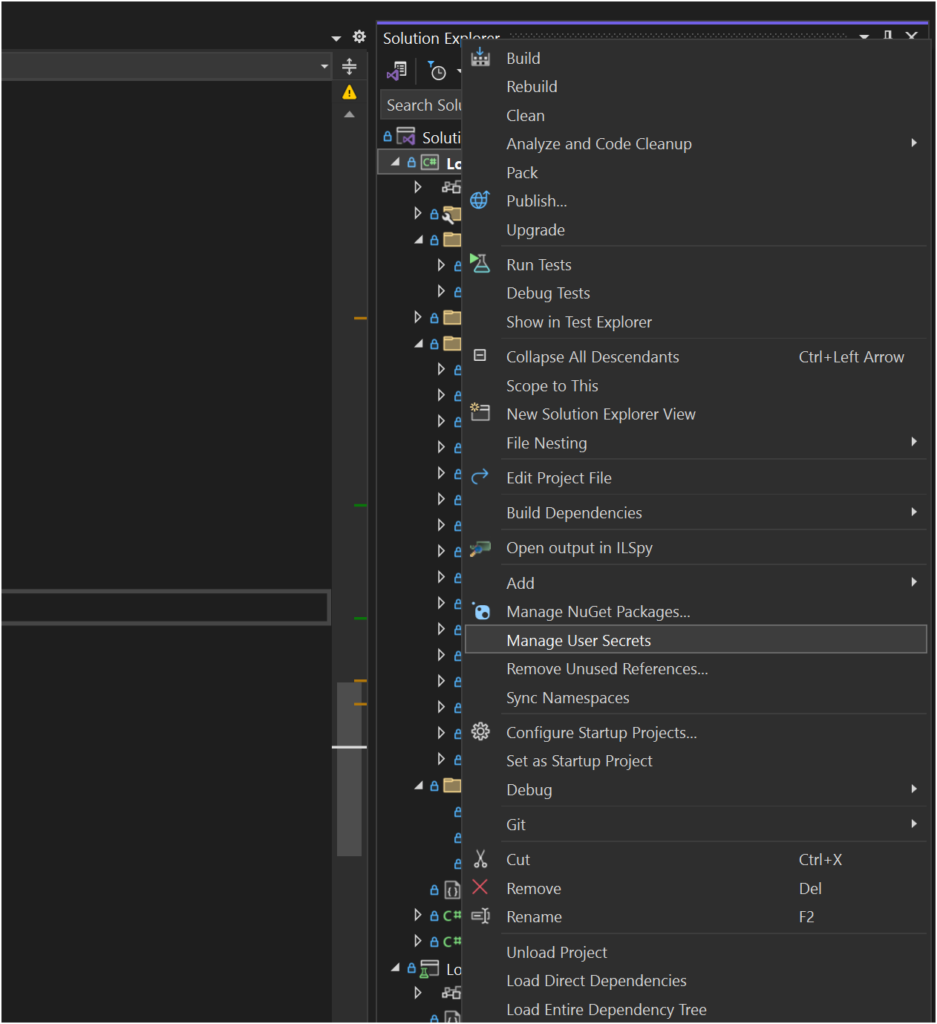
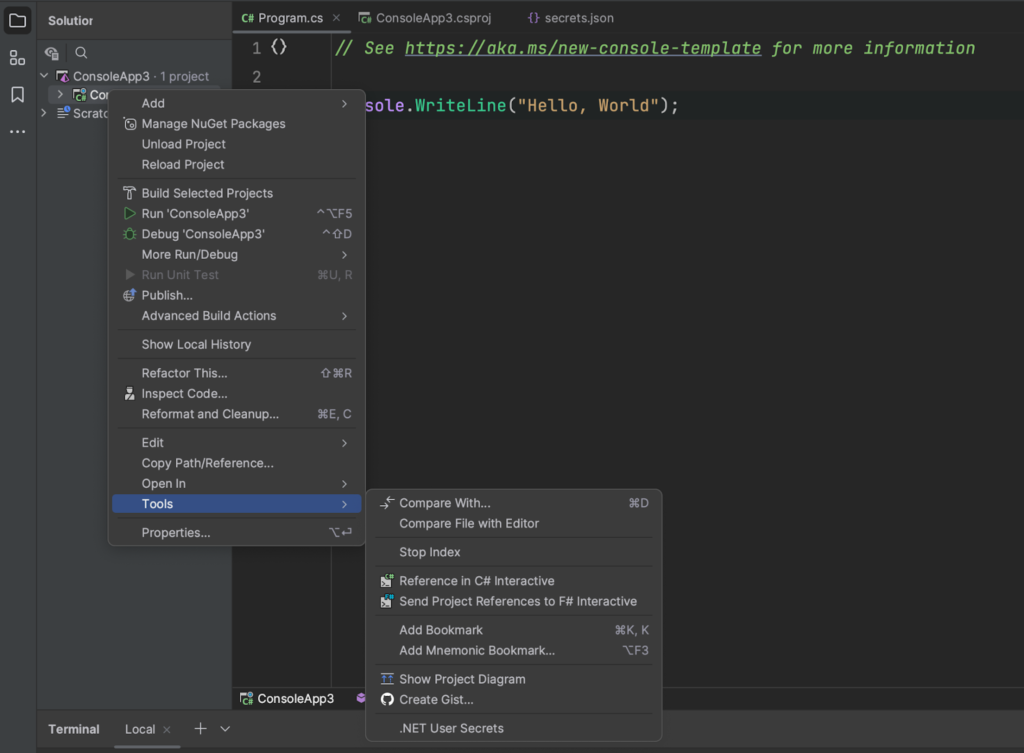
Practical Example: Using Secret Manager in an ASP.NET Core Application
In this section, we’ll walk through a practical example of how to use the Secret Manager tool within an ASP.NET Core application. Previously, I demonstrated how to write a file to Azure Blob Storage locally using Azurite. If you’re interested, you can read about it in detail at this link.
Installing Necessary Package
Before you can use the Secret Manager tool in your ASP.NET Core project, you need to install the Microsoft.Extensions.Configuration.UserSecrets
package. This package provides the necessary functionality to integrate user secrets into your project.
To install the package, run the following command in your terminal or command prompt:
dotnet add package Microsoft.Extensions.Configuration.UserSecrets
Step 1: Initialize Secret Manager
First, let’s initialize the Secret Manager tool in our project. Open a terminal or command prompt in your project directory and run the following command:
dotnet user-secrets init
This command will add a UserSecretsId
element to your project file, which uniquely identifies your project’s secrets.
Step 2: Add Secrets
Next, we’ll add some secrets. For this example, let’s add the Azure Blob Storage connection string. Run the following command:
dotnet user-secrets set "ConnectionStrings:AzureBlobStorage" "your_connection_string_here"
Replace "your_connection_string_here"
with your actual Azure Blob Storage connection string.
Step 3: Access Secrets in Code
To access the secrets in your code, modify your Program.cs
file to read the connection string from the user secrets. Here’s how you can do it:
using Azure.Storage.Blobs;
using Microsoft.Extensions.Configuration;
var config = new ConfigurationBuilder()
.AddUserSecrets<Program>()
.Build();
string? connectionString = config["ConnectionStrings:AzureBlobStorage"];
var blobServiceClient = new BlobServiceClient(connectionString);
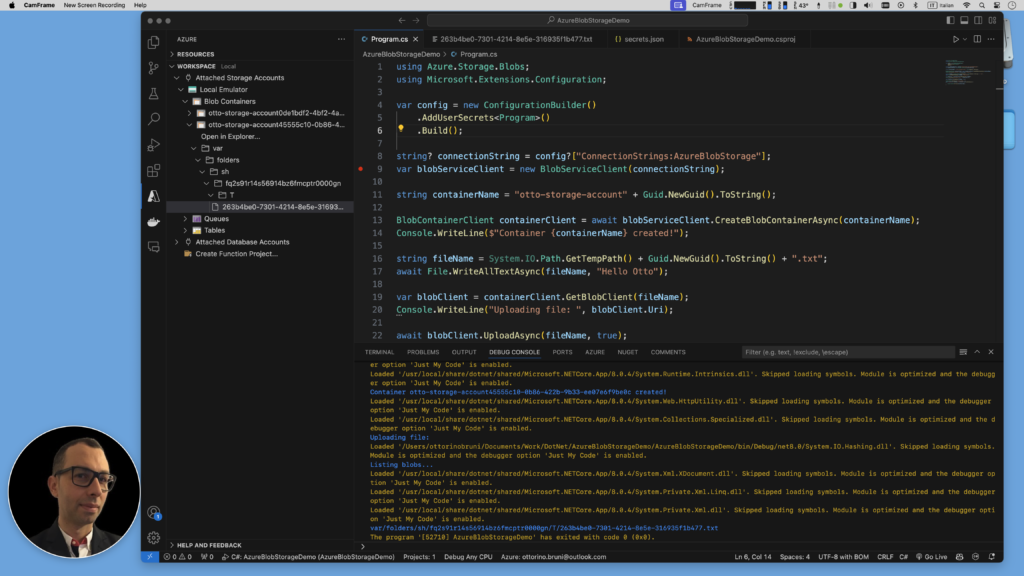
How the Secret Manager Tool Works
The Secret Manager tool is designed to simplify the management of sensitive data during the development of ASP.NET Core applications. It abstracts away the implementation details, allowing you to focus on using the tool without needing to understand where and how the values are stored.
Storage Details
The values managed by the Secret Manager tool are stored in a JSON file located in the user profile folder of the local machine. This file contains the key-value pairs of your secrets.
- Windows: The file is located at
%APPDATA%\Microsoft\UserSecrets\<user_secrets_id>\secrets.json
- Linux / macOS: The file is located at
~/.microsoft/usersecrets/<user_secrets_id>/secrets.json
Tip for macOS Users
When using the Secret Manager tool on macOS, the secrets.json
file is created only the first time you add or set a secret. This is a bit different from Windows, where the file is created during the initialization of the Secret Manager.
To summarize:
- macOS: The
secrets.json
file will be created the first time you set a secret using thedotnet user-secrets set
command. - Windows: The
secrets.json
file is created when you initialize the Secret Manager with thedotnet user-secrets init
command.
This small difference is important to keep in mind to ensure that your secrets are stored correctly and securely during development.
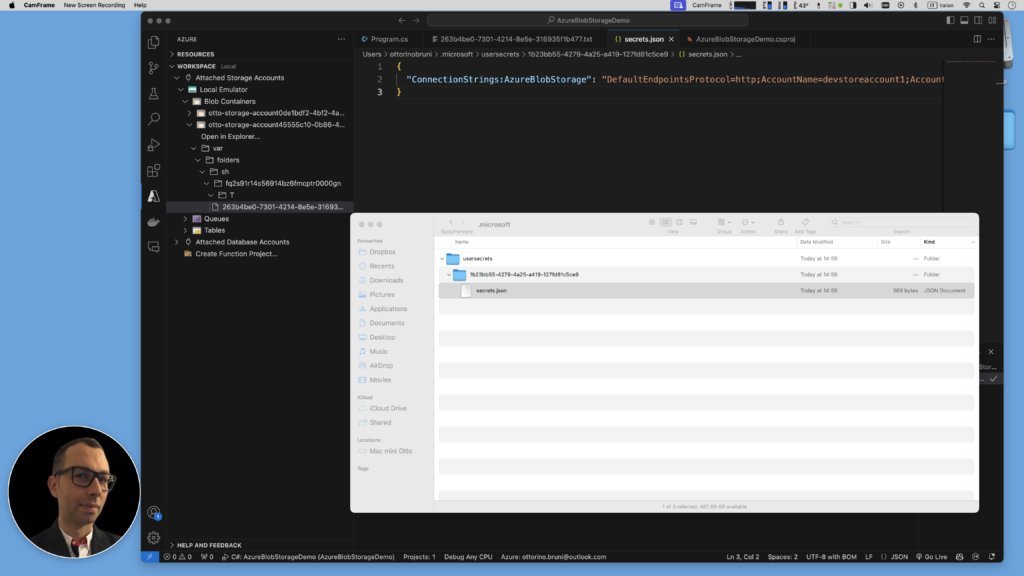
Conclusion: Practicality of Using Secret Manager with .NET
What we’ve learned today? Using the Secret Manager tool with .NET offers a practical way to manage sensitive data like connection strings in ASP.NET Core applications. This tool helps ensure that sensitive information remains secure and isn’t exposed in the source code or accidentally shared on Git repositories.
Key Benefits of Secret Manager:
- Security and Privacy: Secrets are stored securely outside the project directory, preventing accidental exposure in version control systems like Git.
- Ease of Use: Integration with the Secret Manager is straightforward. Once set up, secrets can be easily added, updated, and accessed using simple CLI commands.
- Development Flexibility: Developers can seamlessly switch between local development and production environments without altering code, using different sets of secrets managed through tools like Azure Key Vault.
- Future Integration with Azure Key Vault: As applications scale, integrating with Azure Key Vault enhances security and streamlines management of secrets across environments, ensuring smooth transitions from development to production.
By adopting the Secret Manager tool, developers streamline development processes while adhering to best security practices. Future integration with Azure Key Vault further enhances security and simplifies secret management across different environments, reinforcing the overall security of ASP.NET Core applications.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!