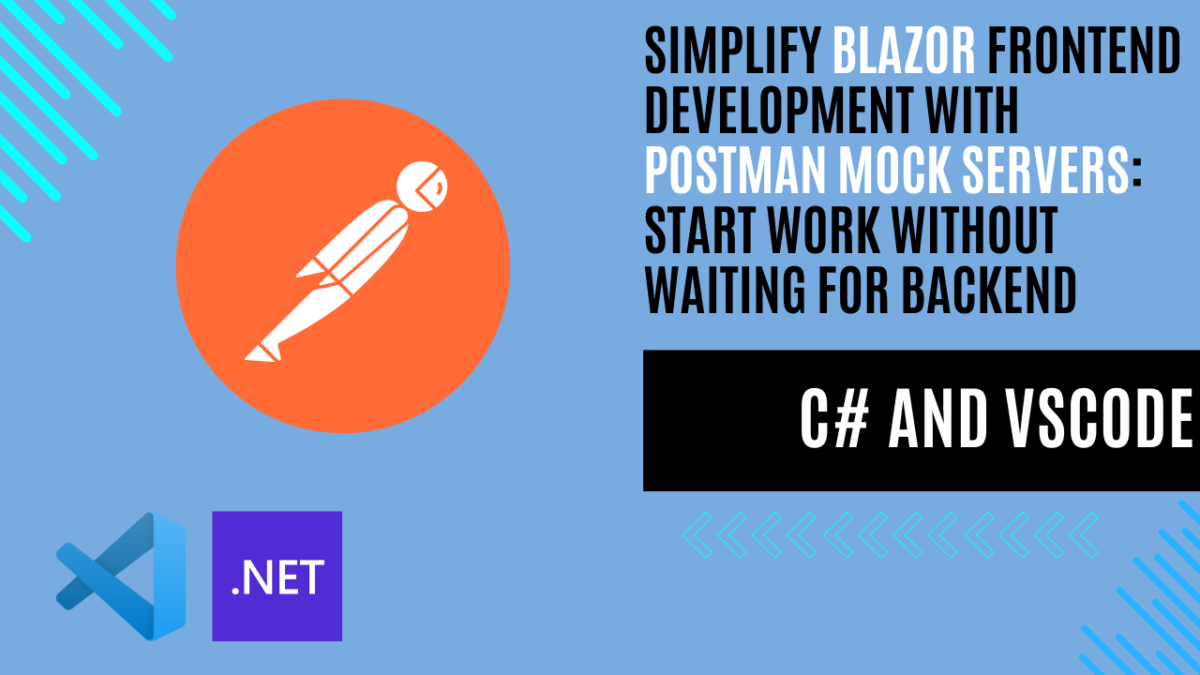
Simplify Blazor Frontend Development with Postman Mock Servers: Start Work Without Waiting for Backend
Introduction
When building modern web applications, APIs are critical for communication between the frontend and backend. However, there are times when backend development lags behind, or you need to start working on the frontend without waiting for the API to be fully implemented. This is where tools like Postman come into play.
In a previous article, I highlighted Postman as one of the Must-have Mac Apps for Software Developers, and for good reason. Postman is widely used for testing and debugging APIs, simplifying the process of sending requests and inspecting responses. But Postman is more than just a tool for API testing—it also includes a powerful feature known as the Postman Mock Server.
A mock server enables you to simulate your API without needing to configure an actual backend server. You can use any HTTP collection to set up a mock server, and when you send a request, Postman returns a real-world response using the data from your collection. This allows you to develop and test your frontend independently from the backend, which is incredibly useful when working with frameworks like Blazor.
But Postman mock servers aren’t just useful during development, they are also valuable when performing End-to-End (E2E) tests. E2E tests focus on testing the complete user workflow, simulating real user interactions. By mocking an external service, you can ensure your E2E tests run smoothly even when the actual API isn’t ready or available. This not only accelerates the development process but also ensures that the entire system, from UI to backend interactions, works as expected.
In this article, we’ll explore how to leverage Postman mock servers in combination with Blazor and C#. Whether you’re a frontend developer simulating APIs to start development early or aiming for robust E2E testing, this guide will walk you through setting up and using mock servers to speed up your workflow.
Why Postman Mock Servers Are Essential for Frontend-Backend Collaboration
In many software development projects, especially those involving agile methodologies, teams work on both frontend and backend components in parallel. However, a recurring challenge arises when frontend developers are blocked, waiting for the backend infrastructure, specifically the API endpoints, to be implemented by backend developers. This dependency can significantly slow down progress and disrupt the flow of feature development.
Frontend development, particularly in modern frameworks like Blazor or React, relies heavily on data from APIs to render components dynamically, manage state, and process user interactions. Without a working API, frontend developers are left with a few options:
- Create placeholder data (which will later need to be replaced with real data),
- Build only the static parts of the UI, or
- Wait until the backend API is ready.
While these methods can help move things forward, they are not ideal for several reasons:
- Placeholder data doesn’t accurately reflect how real-world responses will behave, leading to additional work when the API becomes available.
- Developing only the static parts of the UI can mean the developer isn’t building or testing actual functionality, which is a key part of frontend work.
- Waiting for the backend to be finished wastes time and results in inefficiencies.
The absence of a backend API also makes integration testing nearly impossible, preventing frontend developers from testing their code in a real-world scenario where APIs could return different statuses or error codes (e.g., 404 Not Found, 500 Internal Server Error, 429 Too Many Requests).
This is where tools like Postman Mock Servers become invaluable. By simulating API responses, frontend developers can:
- Work independently of the backend team, using predefined mock responses that mimic the structure and behavior of the future API.
- Simulate different types of responses, including success responses and various error codes (e.g., 400 Bad Request, 404 Not Found), to handle edge cases and error handling logic early in the development process.
- Develop and test dynamic features (such as forms, data tables, charts, etc.) with mock data that closely mirrors what the actual API will return, allowing the frontend to be fully functional even before the backend is ready.
Tutorial: Using Postman Mock Servers with Blazor to Simulate API Responses
Disclaimer: This example is intended for educational purposes. While it provides a good starting point for learning, there are always better ways to write and optimize code and applications. Use this as a base and continually strive to follow best practices and improve your implementations.
Prerequisites
To follow along with this tutorial, you can download the starting example project from the following GitHub repository: DevProxy on GitHub.
The project structure is organized as follows:
- DevProxy.WebApp: Contains the source code for the DevProxy web application, which displays weather forecasts.
- DevProxy.API: Includes the web service for weather data, utilized by the web application.
- DevProxy.sln: Main solution file connecting both the web application and API projects.
Make sure you have the following installed:
- .NET SDK: Download and install the .NET SDK if you haven’t already.
- Visual Studio Code (VSCode): Install Visual Studio Code for a lightweight code editor.
- C# Extension for VSCode: Install the C# extension for VSCode to enable C# support.
Creating a Postman Mock Server
Step-by-Step Instructions:
Log in to Postman: If you don’t have an account, sign up at Postman. Enable menu Mock server in the Settings.
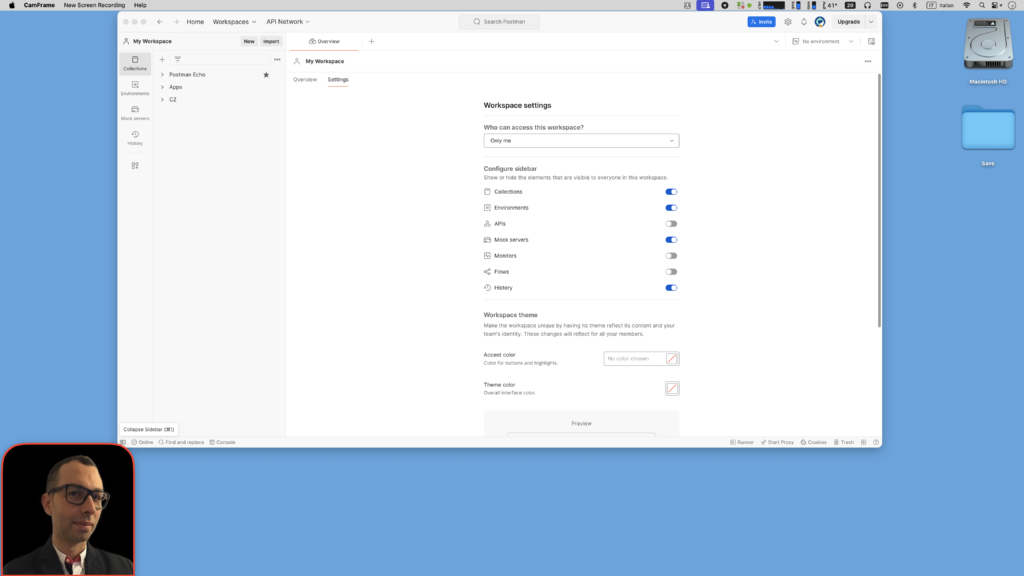
Create a new collection: Go to Collections and click New Collection and enter these requests data:
- Request Method GET,
- Request URL weatherforecast,
- Response Code 200 and add the
- Response Body:
[
{"date":"2024-09-21","temperatureC":3,"summary":"Sweltering","temperatureF":37},
{"date":"2024-09-22","temperatureC":4,"summary":"Balmy","temperatureF":39},
{"date":"2024-09-23","temperatureC":38,"summary":"Mild","temperatureF":100},
{"date":"2024-09-24","temperatureC":44,"summary":"Hot","temperatureF":111},
{"date":"2024-09-25","temperatureC":1,"summary":"Scorching","temperatureF":33}
]
- Request Method GET,
- Request URL weatherforecast?status=error,
- Response Code 400 and add the
- Response Body:
{
"message": "Bad Request: Invalid query parameters."
}
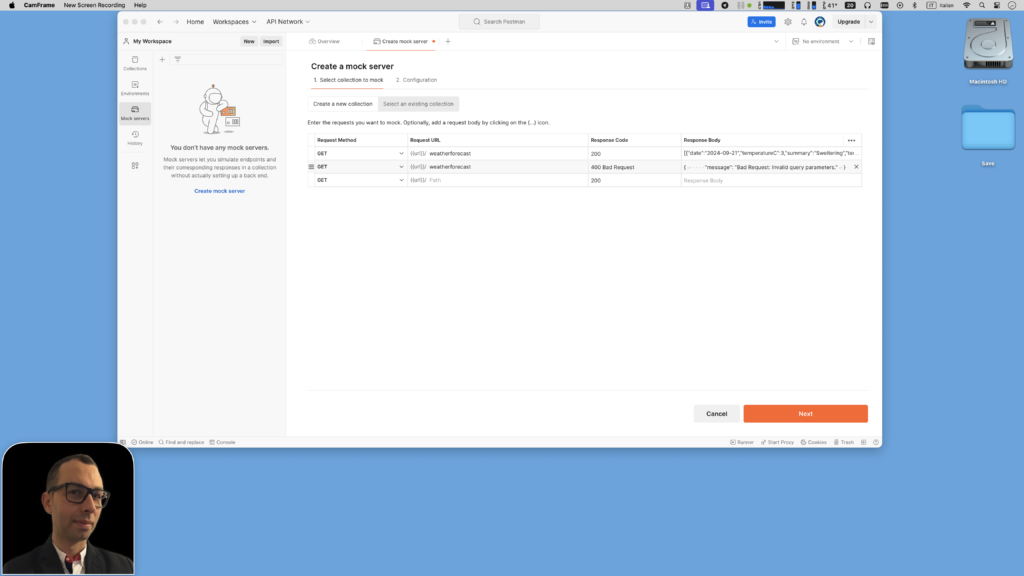
Click Next and add the Mock Server Name “WeatherForecast Mock”.
In this form you can select the Environment and also simulate a fixed network delay. Press Create Mock Server button and we have done.
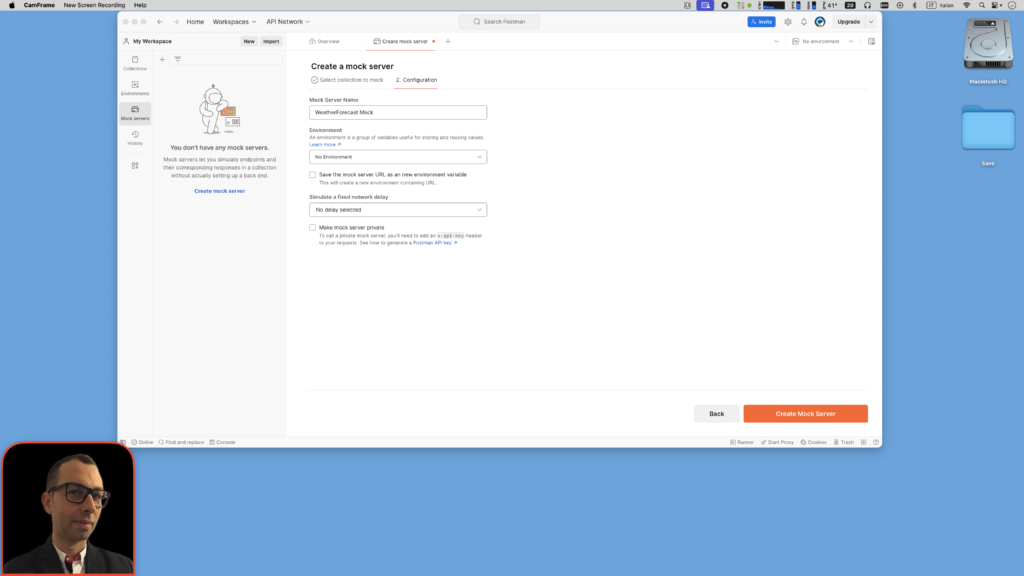
Now if we go to our collections we will find the one we just created named WeatherForecast Mock, let’s try to execute the two created requests and see the results.
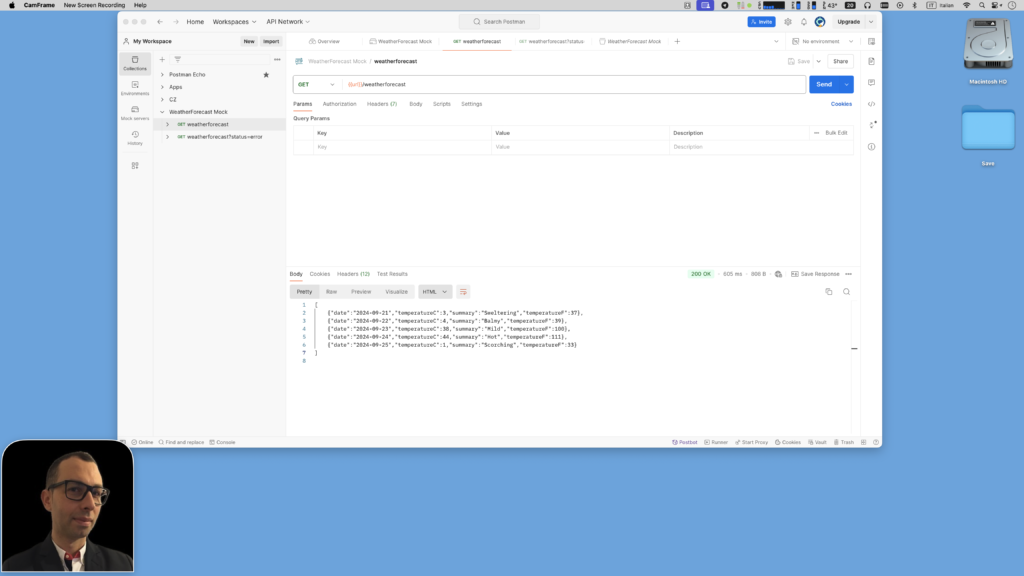
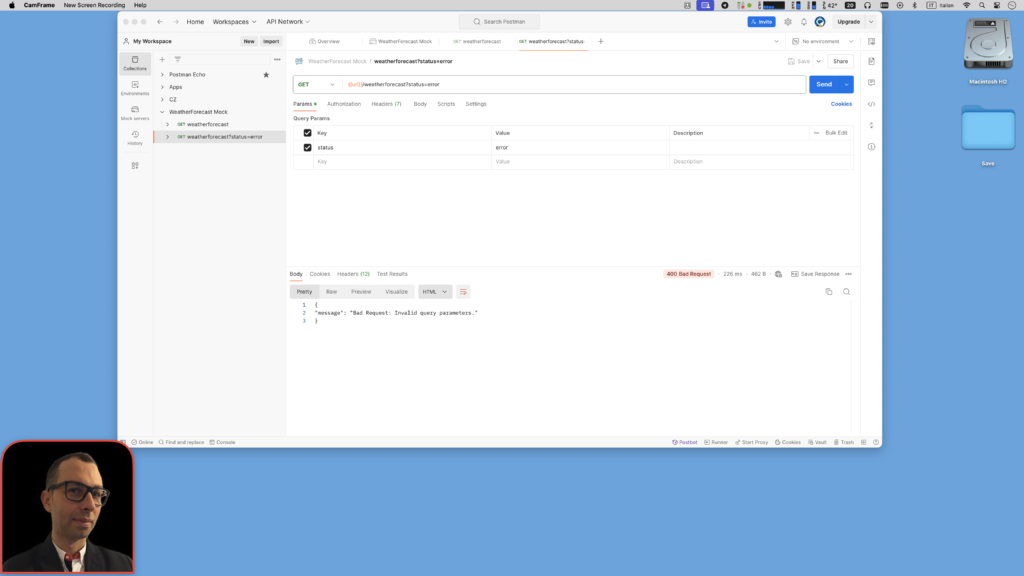
Modifying the Blazor App to Use the Postman Mock Server
Update the Blazor app
Now, you’ll modify the Blazor app to use the mock server instead of the real API.
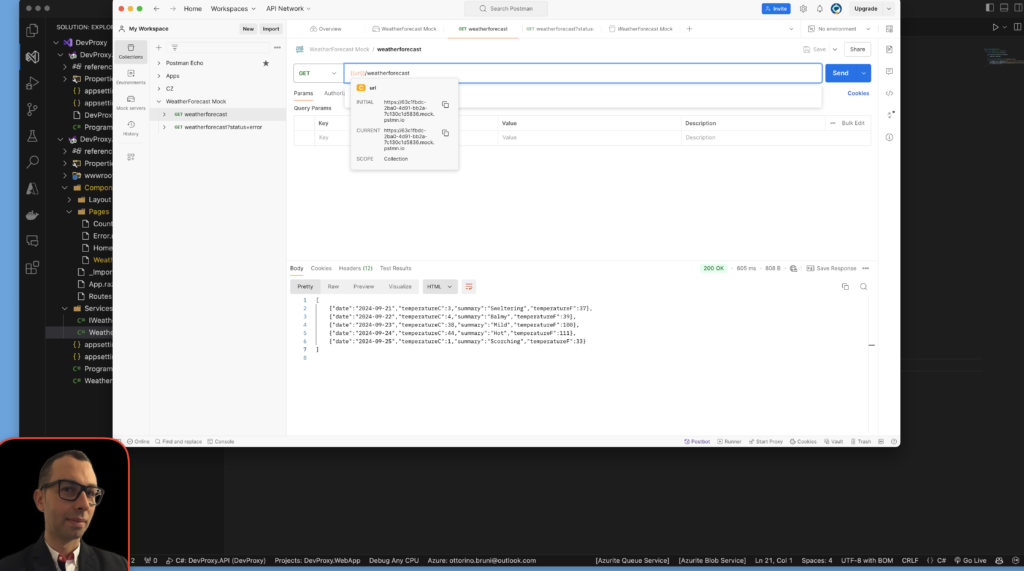
In your WeatherService.cs
class, replace the current API URL with the Postman mock server URL you created:
public async Task<List<WeatherForecast>> GetWeatherForecasts()
{
var response = await _httpClient.GetAsync("https://<mock-url>/weatherforecast");
response.EnsureSuccessStatusCode();
var forecasts = await response.Content.ReadFromJsonAsync<IEnumerable<WeatherForecast>>();
return forecasts?.ToList()!;
}
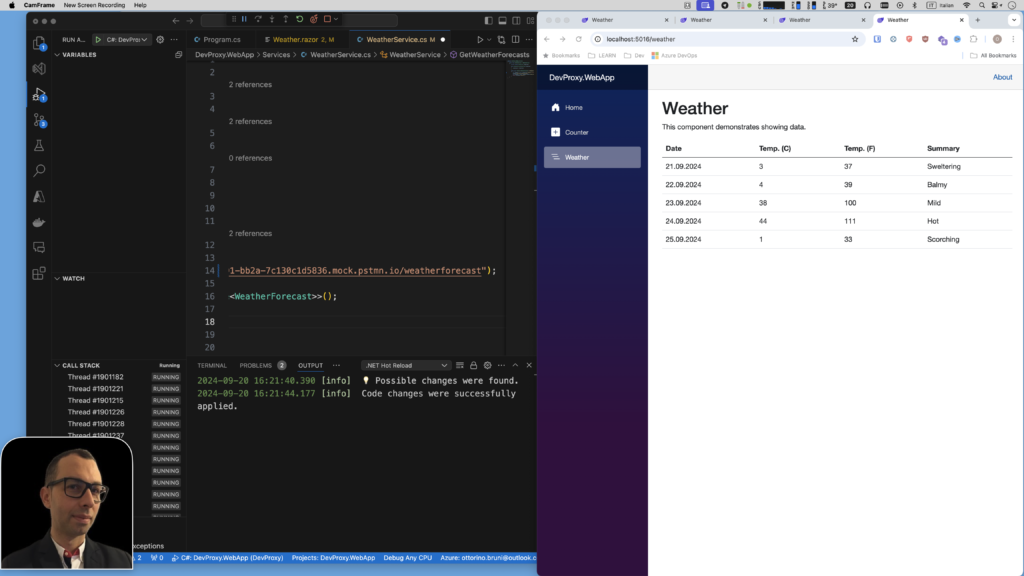
Simulating a 400 Bad Request in Postman Mock Server
In your WeatherService.cs
class, replace the current API URL with the Postman mock server URL you created:
public async Task<List<WeatherForecast>> GetWeatherForecasts()
{
var response = await _httpClient.GetAsync("https://<mock-url>/weatherforecast?status=error");
response.EnsureSuccessStatusCode();
var forecasts = await response.Content.ReadFromJsonAsync<IEnumerable<WeatherForecast>>();
return forecasts?.ToList()!;
}
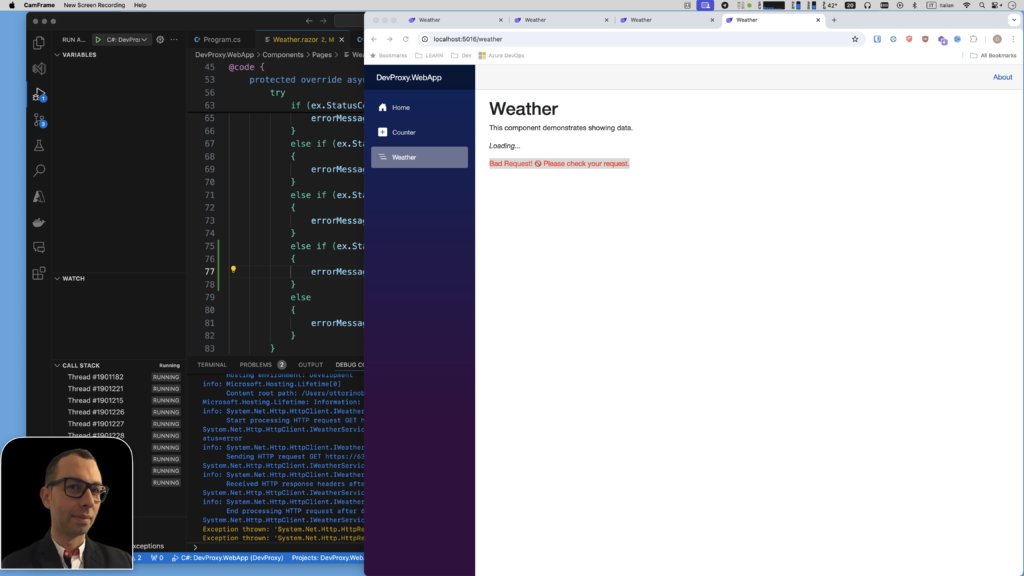
Run the Blazor application
When the Blazor app runs, it will now call the Postman mock server and display the mock data you defined in Postman.
If you’re interested in exploring the source code of this project, you can find it on GitHub.
Conclusion: The Power and Simplicity of Postman for API Development and Testing
Postman has long been a go-to tool for developers when it comes to managing and testing APIs. Its intuitive interface and powerful features allow you to quickly explore, debug, and validate APIs throughout the development process. However, Postman’s capabilities extend far beyond just API management, its Mock Server functionality opens up new possibilities for seamless development and testing workflows.
By leveraging Postman Mock Servers, you can simulate API responses with ease, allowing frontend developers to begin building features without waiting for the backend to be fully implemented. This decoupling of frontend and backend development is invaluable, speeding up the development cycle and enabling more efficient testing of your application’s behavior in real-world scenarios.
In this tutorial, we’ve seen how Postman can help:
- Simulate APIs early in the development process, keeping frontend developers unblocked.
- Create mock responses for various scenarios, such as successful
200 OK
responses or error states like400 Bad Request
, making it easier to handle edge cases in your code. - Test the full user flow with end-to-end (E2E) tests, even when the backend isn’t yet available.
The ability to quickly spin up mock servers makes Postman an essential tool for any developer working with APIs. Whether you’re developing new features, writing tests, or running end-to-end scenarios, Postman Mock Servers provide a simple and powerful solution for both frontend and backend developers to stay productive and aligned.
So, next time you’re facing a bottleneck while waiting for the backend to catch up, give Postman Mock Servers a try, you’ll be amazed at how quickly you can simulate real-world scenarios and keep your project moving forward.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!