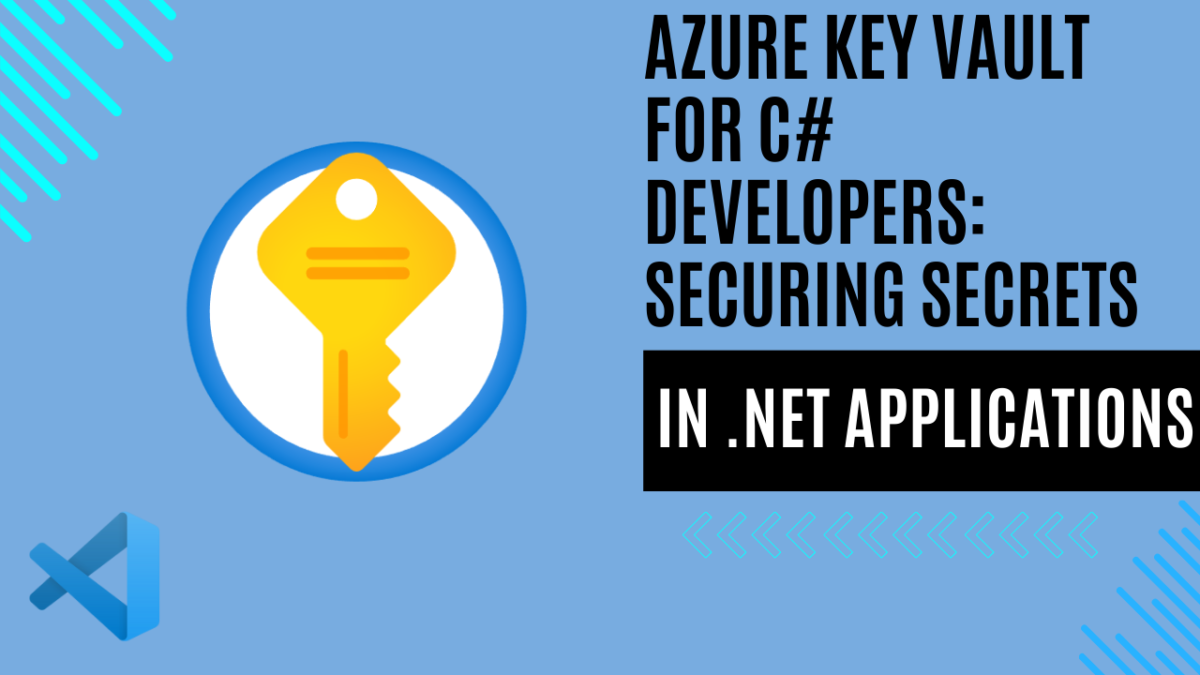
Azure Key Vault for C# Developers: Securing Secrets in .NET Applications
Introducing Azure Key Vault
In the last article, I discussed how to secure and remove connection strings and passwords from your local code using Secret Manager. If you haven’t read it yet, you can check out the previous article here. Today, we’ll dive into Azure Key Vault, explaining what it is and how it simplifies the development of enterprise applications.
What is Azure Key Vault?
Azure Key Vault is a cloud service designed to safeguard cryptographic keys and secrets used by cloud applications and services. It enables secure storage and tight control over access to these sensitive data, offering several advantages for enterprise application development:
- Enhanced Security: Azure Key Vault ensures that secrets, such as API keys, passwords, and certificates, are stored securely and accessed only by authorized applications and users.
- Centralized Management: With Azure Key Vault, you can manage secrets and keys centrally, reducing the complexity and risk associated with handling sensitive information in multiple locations.
- Scalability: As your application grows, Azure Key Vault scales seamlessly, supporting increased demand and maintaining performance.
- Compliance: Using Azure Key Vault helps meet compliance requirements by providing detailed access logs and audit trails, essential for regulatory standards.
In the following sections, we’ll cover how to:
- Set Up Azure Key Vault: Create and configure a Key Vault in the Azure portal.
- Integrate with .NET Applications: Access secrets from Azure Key Vault within your ASP.NET Core applications.
Azure Key Vault: Key Features and Benefits
Containers Supported:
- Vaults: Store software and HSM-backed keys, secrets, and certificates.
- Managed HSM Pools: Only support HSM-backed keys.
Primary Functions:
- Secrets Management: Secure storage and controlled access to tokens, passwords, certificates, API keys, and other secrets.
- Key Management: Create and control encryption keys used to secure data.
- Certificate Management: Provision, manage, and deploy SSL/TLS certificates for Azure and internal resources.
Service Tiers:
- Standard: Encrypts with a software key.
- Premium: Includes hardware security module (HSM)-protected keys.
Key Benefits:
- Centralized Secrets Storage: Securely store application secrets in Key Vault, accessed via URIs instead of embedding in code.
- Secure Access: Authentication via Microsoft Entra ID and authorization via Azure RBAC or Key Vault access policies.
- Access Monitoring: Enable logging to monitor and control access, with options to archive, stream, or send logs to Azure Monitor.
- Simplified Administration:
- No need for in-house HSM knowledge.
- Scales with usage spikes.
- Replicates data for high availability.
- Standard Azure administration through portal, CLI, and PowerShell.
- Automates certificate enrollment and renewal from Public CAs.
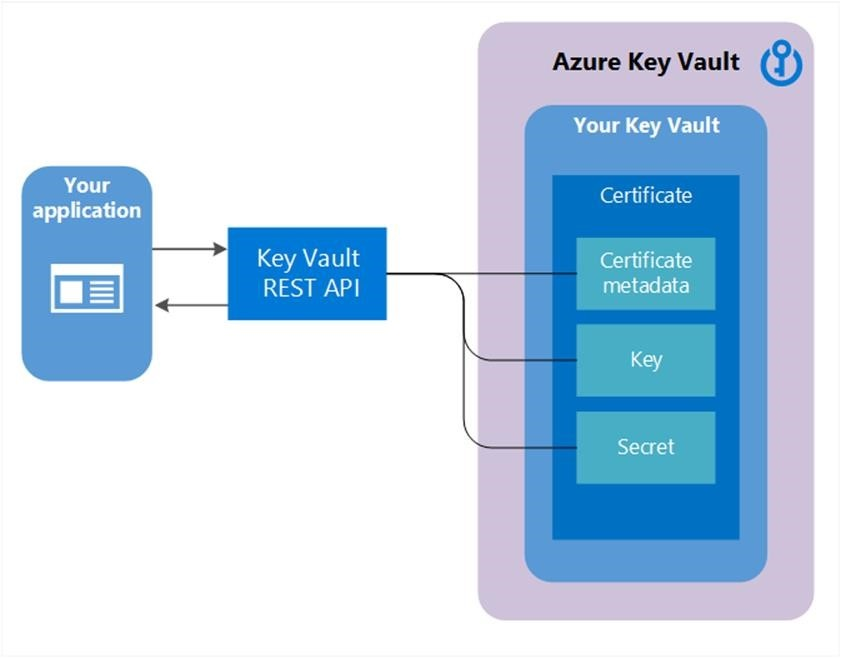
Creating an Azure Key Vault and Adding a Secret
Step 1: Create an Azure Key Vault
- Sign in to the Azure Portal: Go to the Azure Portal and sign in with your Azure account.
- Create a Key Vault:
- Navigate to “Create a resource” in the upper left corner.
- Search for “Key Vault” and select it.
- Click “Create.”
- Configure Key Vault Settings:
- Subscription: Select your subscription.
- Resource Group: Select an existing resource group or create a new one.
- Key Vault Name: Provide a unique name for your Key Vault.
- Region: Choose the region closest to your location.
- Pricing Tier: Choose between Standard and Premium. (Standard is usually sufficient for most use cases.)
- Click “Review + create,” then “Create” after the validation passes.
- Access Your Key Vault: Once the deployment is complete, go to “All resources” and select your newly created Key Vault.
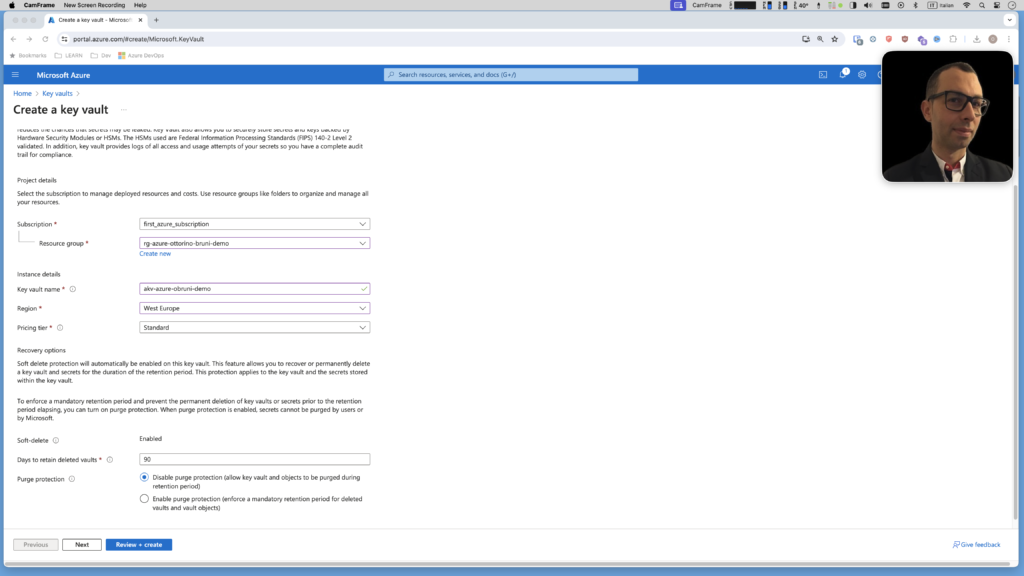
Step 2: Add a Secret to Your Key Vault
- Navigate to Secrets:
- In your Key Vault, navigate to the “Secrets” section in the left-hand menu.
- Click on “+ Generate/Import.”
- Create a Secret:
- Upload options: Select “Manual” for manually entering the secret value.
- Name: Provide a name for your secret (e.g., “MySecret”).
- Value: Enter the secret value (e.g., a connection string or password).
- Click “Create” to add the secret to your Key Vault.
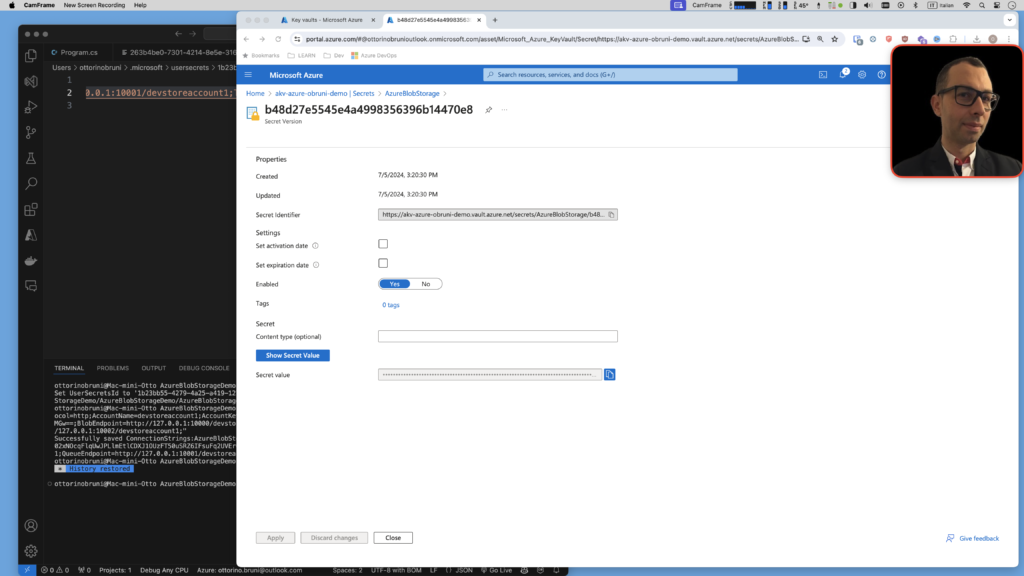
Practical Example: Using Azure Key Vault in a .NET Application
In this section, we’ll walk through a practical example of how to use the Azure Key Vault within a .NET application. Previously, I demonstrated how to write a file to Azure Blob Storage locally using Azurite and User Secrets. If you’re interested, you can read about it in detail at this link or at this link.
Now, let’s reuse the same code but instead of retrieving the connection string from user secrets, we’ll fetch it from Azure Key Vault.
To modify your code to fetch the connection string from Azure Key Vault instead of user secrets, follow these steps:
- Install Required Packages: Ensure you have installed the necessary NuGet packages:
dotnet add package Azure.Identity
dotnet add package Azure.Security.KeyVault.Secrets
- Update Code: Replace the code that retrieves the connection string from user secrets with code that retrieves it from Azure Key Vault using
SecretClient
fromAzure.Security.KeyVault.Secrets
.
using Azure.Identity; using Azure.Security.KeyVault.Secrets; using Azure.Storage.Blobs; var keyVaultName = "akv-azure-obruni-demo"; var secretName = "AzureBlobStorage"; var client = new SecretClient(new Uri($"https://{keyVaultName}.vault.azure.net/"), newDefaultAzureCredential(includeInteractiveCredentials: true)); KeyVaultSecret secret = await client.GetSecretAsync(secretName); string connectionString = secret.Value; var blobServiceClient = new BlobServiceClient(connectionString); string containerName = "otto-storage-account" + Guid.NewGuid().ToString(); BlobContainerClient containerClient = await blobServiceClient.CreateBlobContainerAsync(containerName); Console.WriteLine($"Container {containerName} created!"); string fileName = System.IO.Path.GetTempPath() + Guid.NewGuid().ToString() + ".txt"; await File.WriteAllTextAsync(fileName, "Hello Otto"); var blobClient = containerClient.GetBlobClient(fileName); Console.WriteLine("Uploading file: ", blobClient.Uri); await blobClient.UploadAsync(fileName, true); System.Console.WriteLine("Listing blobs..."); await foreach(var blobItem in containerClient.GetBlobsAsync()) { System.Console.WriteLine(blobItem.Name); }
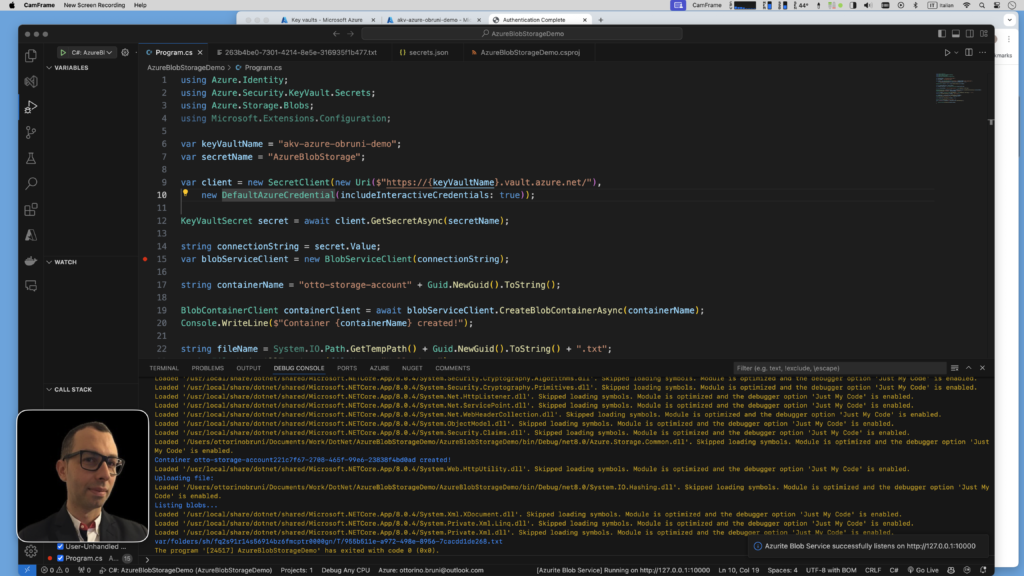
These lines of code initialize a SecretClient
to connect to Azure Key Vault and use DefaultAzureCredential
for authentication. Then, they fetch a secret (secretName
) from the specified Azure Key Vault (keyVaultName
). This approach ensures secure access to secrets without exposing credentials in your application code.
Best Practices for Using Azure Key Vault
Remember, the code provided is purely educational and should never be used in a production environment to maintain security and compliance.
Azure Key Vault offers robust security for sensitive information.
- Best practices include using managed identities to authenticate applications without exposing credentials.
- Implement Azure RBAC for access control, and regularly rotate secrets with versioning for security.
- Enable auditing and monitoring to track access and detect anomalies.
- Securely configure Key Vault by avoiding hardcoded URIs and using environment variables or Azure App Configuration.
These practices ensure Azure Key Vault enhances security and compliance, mitigating risks in managing sensitive data for Azure solutions.
Conclusion: Secure Secret and Key Management with Azure Key Vault
Combining User Secrets for local development and Azure Key Vault for production provides numerous benefits. Azure Key Vault ensures secure storage and controlled access for secrets, certificates, and keys, enhancing overall application security. It simplifies management with straightforward integration and offers benefits such as:
- Enhanced Security: Safely store and tightly control access to sensitive information like passwords, API keys, certificates, and encryption keys.
- Simplified Management: Seamless integration with Azure services and applications, ensuring easy access and updates to secrets.
- Compliance and Auditing: Meet regulatory requirements with built-in auditing, logging, and access control features.
By leveraging User Secrets during development and Azure Key Vault in production, developers maintain a consistent security posture while simplifying secret management across environments.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!