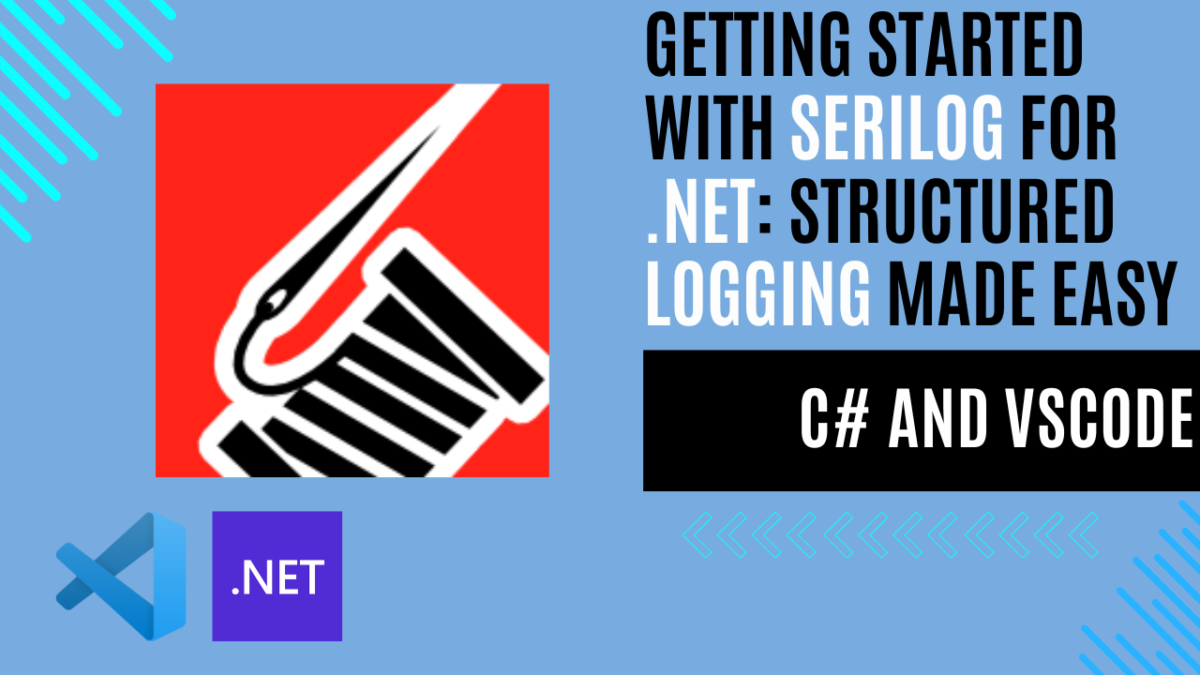
Getting Started with Serilog for .NET and C#: Structured Logging Made Easy
Introduction to Logging and Why It Matters in .NET Applications
In modern software development, logging plays an essential role in monitoring, debugging, and maintaining applications. Logging is the process of recording events or messages from an application’s execution, which can provide critical insights for developers and administrators alike. Whether you’re building a small utility or a large-scale enterprise solution, implementing effective logging can make the difference between quickly identifying a bug and spending hours (or even days) trying to reproduce and diagnose it.
In .NET applications, logging serves several key purposes:
- Debugging and Troubleshooting: Logs provide a record of an application’s actions and errors, allowing developers to diagnose issues without needing to replicate them directly. For example, if a user reports a failure, logs can reveal the specific actions that led to the error.
- Monitoring and Performance Optimization: Logs help monitor application health, showing performance bottlenecks or inefficiencies. For instance, if response times start to slow, logs can help pinpoint where delays occur in the code.
- Auditing and Compliance: In regulated industries, it’s essential to keep records of certain actions, especially those related to user data or sensitive transactions. Logging provides a way to track these events and ensure compliance with regulations.
- Operational Insights: With structured and well-organized logs, developers and operations teams gain insights into user behavior, application usage, and system health, which can help guide future development decisions.
Traditional logging usually consists of unstructured text, which is useful but limited. As applications scale, especially with modern architectures like microservices, the need for structured logging arises. Structured logging organizes data into key-value pairs, making it easier to filter, analyze, and visualize logs across complex, distributed systems.
What is Serilog? An Overview of Its Features and Benefits
Serilog is a popular, open-source logging library for .NET, known for its structured logging capabilities and ease of use. Unlike traditional logging libraries that focus on plain text logs, Serilog allows you to log structured data, which is ideal for capturing contextual information. This makes it perfect for complex .NET applications, where structured logs help make sense of application behavior across distributed or asynchronous systems.
Here’s a quick overview of what makes Serilog a standout choice for .NET developers:
- Structured Data: Serilog lets you log properties with your messages. Instead of logging raw text like
"Error processing order 123"
, you can log structured data, such as{ OrderId: 123, Status: "Error" }
. This approach improves readability and searchability, especially in data-rich logs. - Flexible Configuration: Serilog is versatile and can be set up in a variety of ways. You can configure it directly in code or use a configuration file (e.g.,
appsettings.json
) for easy updates without touching the codebase. This flexibility makes it easy to adjust logging behavior according to environment or application needs. - Wide Range of Sinks: Serilog has a robust ecosystem of sinks, which are outputs for your log data. By default, it supports basic outputs like the console and files, but you can also log to databases, cloud services, or specialized tools (such as Seq, Elasticsearch, or Application Insights) to store, analyze, or visualize log data.
- Compatibility: Serilog is compatible with all recent .NET versions, including .NET Core, .NET 5/6, and later, making it a go-to choice for both legacy and modern .NET applications. Additionally, it integrates seamlessly with popular .NET frameworks and libraries, like ASP.NET Core, which makes it easy to add logging to web applications, APIs, and other services.
- Extensibility and Customization: With Serilog, you can use enrichers to add custom data to each log event (such as user IDs, request information, or geographic data). You can also define custom sinks or use plugins to extend Serilog’s functionality according to your specific needs.
In short, Serilog’s combination of structured logging, flexibility, and rich ecosystem makes it an ideal choice for developers who need a powerful, maintainable logging solution in .NET. Whether you’re working on a simple application or a distributed microservices architecture, Serilog simplifies the process of capturing and managing logs in a way that scales with your application’s complexity.
Setting Up Serilog in .NET Applications
To get started with Serilog in a .NET project, we first need to install a few NuGet packages. These packages provide the core Serilog functionality, along with optional “sinks” for writing logs to different destinations (like the console or a file). Each package has a specific role, allowing you to customize Serilog according to your application’s needs.
Here’s a breakdown of the essential packages to install and what each one does:
dotnet add package Serilog
dotnet add package Serilog.Sinks.Console
dotnet add package Serilog.Sinks.File
dotnet add package Serilog.Extensions.Logging
dotnet add package Serilog.Settings.Configuration
Here’s a concise summary of each package’s purpose:
- Serilog: The core library for creating and managing structured logs.
- Serilog.Sinks.Console: Enables logging to the console, useful for real-time feedback during development.
- Serilog.Sinks.File: Allows logging to a file, essential for keeping a log history in production.
- Serilog.Extensions.Logging: Integrates Serilog with ASP.NET Core’s logging system, replacing the default provider for better structured logging in web applications.
- Serilog.Settings.Configuration: Allows you to configure Serilog using settings from a configuration file, such as appsettings.json
With these packages, you’ll be able to configure and use Serilog to log messages to both the console and files, and fully integrate it within ASP.NET Core if needed.
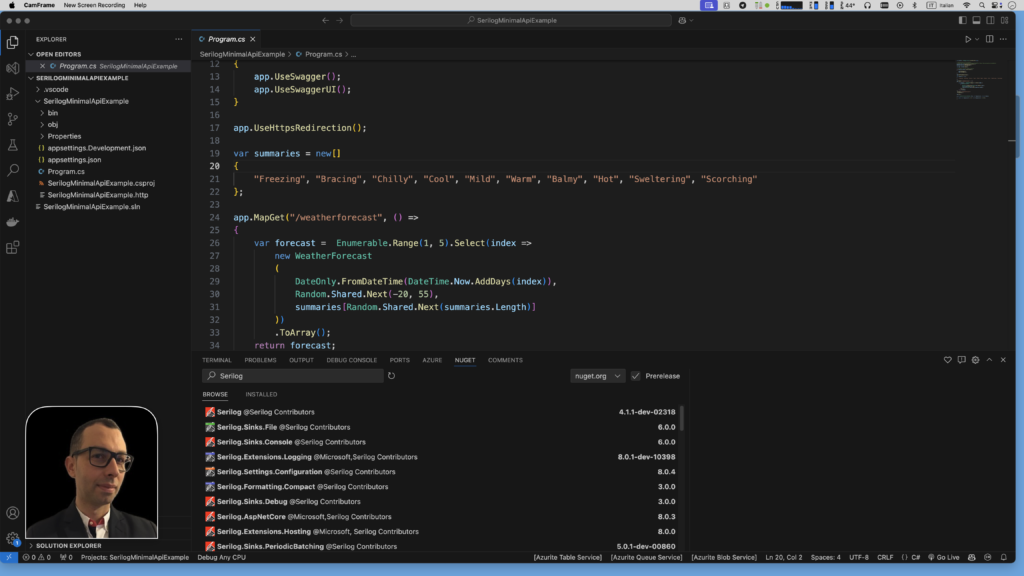
Configuring Serilog: Code-Based vs. Configuration File Setup
Configuring Serilog: Fluent API-Based vs. Configuration File Setup
When setting up logging with Serilog, you have two main configuration options: Fluent API-based setup and configuration file-based setup. Each approach has its advantages. Configuring Serilog directly in code gives you full control and is ideal for quick setups or for settings that need to change dynamically. On the other hand, using a configuration file (like appsettings.json
) allows for a more flexible and manageable approach, especially in production environments, where changing settings without redeploying code is a significant advantage.
Fluent API-Based Configuration
In a Fluent API-based setup, you configure Serilog directly in your application’s code, which gives you more control and flexibility, especially for quick setups or for dynamically changing settings.
// Configure Serilog
Log.Logger = new LoggerConfiguration()
.MinimumLevel.Debug() // Set the minimum log level
.WriteTo.Console() // Console sink for development
.WriteTo.File("logs/log.txt", // File sink with specified path
rollingInterval: RollingInterval.Day) // Daily log rotation
.CreateLogger();
try
{
Log.Information("Starting application");
}
catch (Exception ex)
{
Log.Fatal(ex, "Application start-up failed");
}
finally
{
Log.CloseAndFlush(); // Ensure all logs are flushed before exit
}
Configuration File-Based Setup (appsettings.json)
Using a configuration file is ideal for managing settings without changing code. This allows you to adjust log settings without recompiling your application.
{
"Serilog": {
"MinimumLevel": {
"Default": "Debug"
},
"WriteTo": [
{ "Name": "Console" },
{
"Name": "File",
"Args": {
"path": "logs/log.txt",
"rollingInterval": "Day"
}
}
]
}
}
// Read configuration from appsettings.json
var configuration = new ConfigurationBuilder()
.AddJsonFile("appsettings.json")
.Build();
// Load Serilog settings from appsettings.json
Log.Logger = new LoggerConfiguration()
.ReadFrom.Configuration(configuration)
.CreateLogger();
try
{
Log.Information("Starting application");
}
catch (Exception ex)
{
Log.Fatal(ex, "Application start-up failed");
}
finally
{
Log.CloseAndFlush(); // Ensure all logs are flushed before exit
}
Example: Using Serilog with .NET Minimal API
In this example, we used a configuration file for Serilog in a .NET Minimal API, allowing you to manage logging settings without changing code. This approach provides the flexibility to update log levels, add sinks, or adjust settings simply by modifying appsettings.json
. This setup is an excellent starting point for adding structured logging to your .NET applications.
Disclaimer: This example is intended for educational purposes only. While it demonstrates key concepts, there are more efficient ways to write and optimize this code in real-world applications. Consider this a starting point for learning, and always aim to follow best practices and refine your implementation.
Prerequisites
Before starting, make sure you have the following installed:
- C# Extension for VSCode: Install the C# extension for VSCode to enable C# support.
- .NET SDK: Download and install the .NET SDK if you haven’t already.
- Visual Studio Code (VSCode): Install Visual Studio Code for a lightweight code editor.
Step 1: Create a New .NET Minimal API Project
Open Terminal in Visual Studio Code or your preferred terminal application and run the following commands:
dotnet new web -o SerilogMinimalApiExample
cd SerilogMinimalApiExample
Step 2: Install Serilog and Configuration Packages
Add the necessary Serilog packages by running the following commands:
dotnet add package Serilog
dotnet add package Serilog.Sinks.Console
dotnet add package Serilog.Sinks.File
dotnet add package Serilog.Settings.Configuration
dotnet add package Serilog.Extensions.Hosting
Step 3: Configure Serilog in appsettings.json
In the project directory, locate the appsettings.json
file. Add the following Serilog configuration to it:
{
"Serilog": {
"MinimumLevel": {
"Default": "Information"
},
"WriteTo": [
{ "Name": "Console" },
{
"Name": "File",
"Args": {
"path": "logs/log.txt",
"rollingInterval": "Day"
}
}
]
}
}
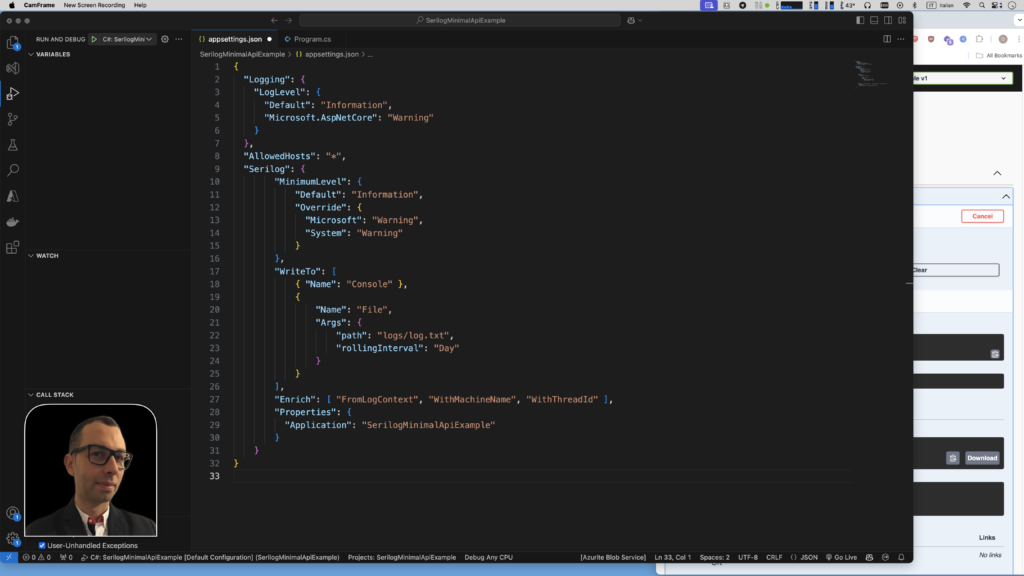
Step 4: Set Up Serilog in Program.cs
Open Program.cs
and modify it to initialize Serilog using the configuration file.
using Serilog;
var builder = WebApplication.CreateBuilder(args);
// Configure Serilog from appsettings.json
builder.Host.UseSerilog((context, services, configuration) =>
{
configuration.ReadFrom.Configuration(context.Configuration);
});
// Add services to the container.
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.MapGet("/log", (ILogger<Program> logger) =>
{
// Logging messages at different levels
logger.LogInformation("Operation completed: The user was successfully retrieved from the database.");
logger.LogWarning("Caution: The user data might be outdated or incomplete.");
logger.LogError("Error encountered: Failed to update user details due to database conflict.");
logger.LogCritical("Critical issue: User data is corrupted and may cause system instability.");
return Results.Ok("Log messages have been written to the log file.");
})
.WithName("GetLog")
.WithOpenApi();
app.Run();
Step 5: Run the Application and Check the Logs
Run the application with:
dotnet run
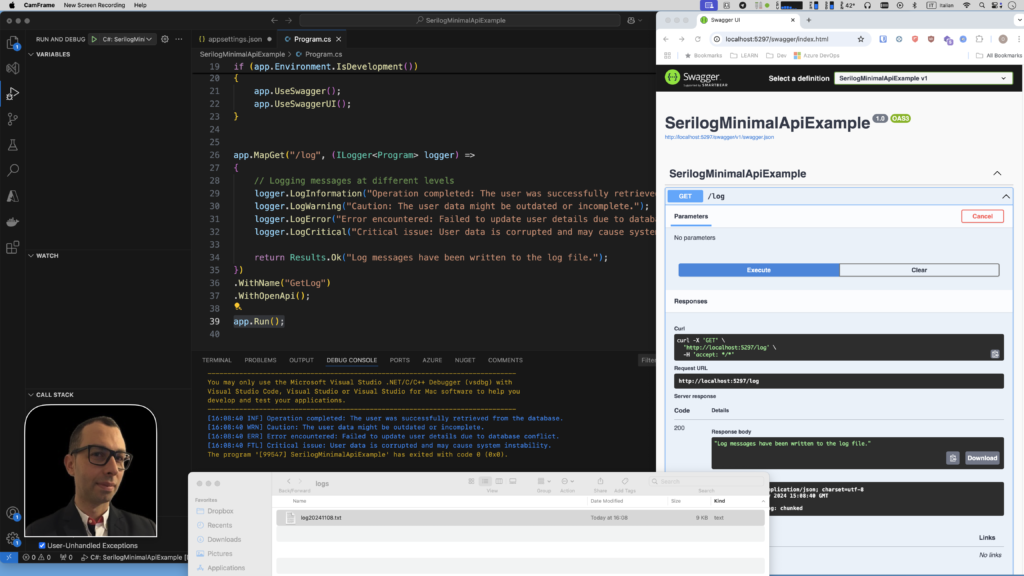
Updated appsettings.json.
Here’s a new configuration for your app based on your template:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"Serilog": {
"MinimumLevel": {
"Default": "Information",
"Override": {
"Microsoft": "Warning",
"System": "Warning"
}
},
"WriteTo": [
{ "Name": "Console" },
{
"Name": "File",
"Args": {
"path": "logs/log.txt",
"rollingInterval": "Day"
}
}
],
"Enrich": [ "FromLogContext", "WithMachineName", "WithThreadId" ],
"Properties": {
"Application": "SerilogMinimalApiExample"
}
}
}
- First configuration:
- Only configures Serilog with a default log level of
Information
and log sinks (console and file).
- Only configures Serilog with a default log level of
- Second configuration:
- Adds
Logging
section to configure log levels (Microsoft.AspNetCore
set toWarning
). - Includes
AllowedHosts
for security. - Overrides log levels for
Microsoft
andSystem
. - Adds log enrichment (
FromLogContext
,WithMachineName
,WithThreadId
) and a customApplication
property.
- Adds
Database Sink: Storing Logs in a Database
A Database Sink outputs log events directly to a database, offering a centralized location for log data. This setup is particularly useful when you need to query, analyze, or aggregate logs using SQL.
Examples of database sinks include:
Serilog.Sinks.MSSqlServer
for logging to SQL Server.Serilog.Sinks.PostgreSQL
for PostgreSQL.Serilog.Sinks.MySql
for MySQL.
Benefits:
- Centralized log storage for easier management.
- SQL querying capabilities for advanced log analysis.
Considerations:
- Writing logs to a database may be slower than other sinks, potentially impacting database performance and storage.
- Ensure that logging frequency does not cause unnecessary strain on the database.
Use Seq for Local Development
Seq is a self-hosted log server designed for searching, analyzing, and alerting on structured log data. It is free for local development and provides advanced search and filtering options, making it an excellent choice for developers.
Writing Logs to Seq
Logs written to Seq are captured as structured JSON data, making them easier to search, filter, and analyze. This can significantly improve your ability to troubleshoot and understand application behavior.
Installing Seq Locally
To get started with Seq, you can download and install it on your local machine:
- Visit the Seq download page.
- Download the
.msi
installer for Windows or follow the installation guide for other systems. - Once installed, you can start logging to Seq, accessing your logs through a web interface, and using advanced search features for faster log analysis.
Seq’s ability to handle structured data and offer real-time searching can greatly enhance the logging experience, especially for developers working on complex systems.
Conclusion: Benefits of Using Serilog for .NET Logging
As a developer with years of experience, I can confidently say that there’s never been a project where I didn’t rely on Serilog for logging. It’s become such an integral part of my development workflow that I can’t imagine working without it. One of the biggest advantages of using Serilog is how easy it is to set up and expand. Whether you’re building a small project or a complex distributed system, Serilog provides the flexibility to fit your needs. You can quickly start logging with minimal configuration, and as your requirements grow, you can easily add sinks, enrich logs, and change log levels all without disrupting your application.
For us developers, logging is absolutely critical. It’s not just about tracking errors—it’s about gaining insights into how our applications are performing. Structured logging allows us to log events in a way that machines can easily process and search. Unlike traditional logs, which can become hard to navigate, structured logs follow a consistent format and provide richer context, which makes it easier to spot patterns and identify issues.
In conclusion, Serilog is a powerful, flexible, and essential tool for logging in .NET applications. Whether you’re working on a simple app or a complex microservices architecture, Serilog’s structured approach to logging offers clarity, efficiency, and the ability to evolve as your project grows.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!
Discover CodeSwissKnife, your all-in-one, offline toolkit for developers!
Click to explore CodeSwissKnife