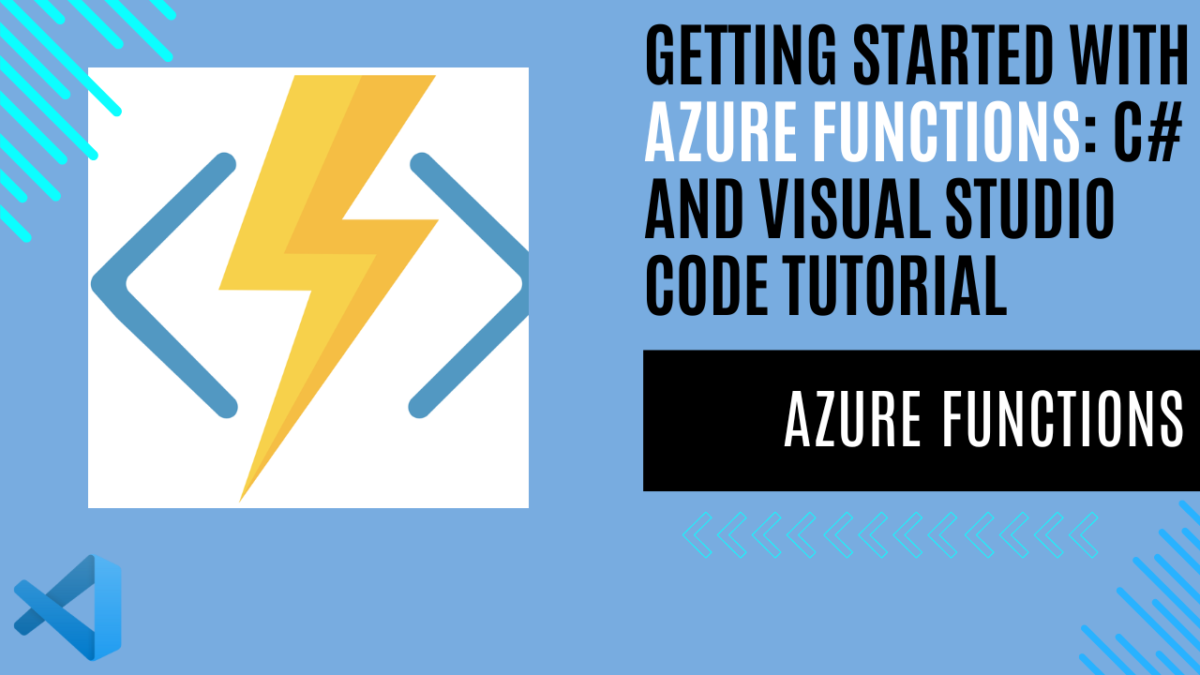
Getting Started with Azure Functions: C# and Visual Studio Code Tutorial
Introduction
If I had to choose my favorite Azure cloud service, I would definitely choose Azure Functions. Why? Because it is simply one of the easiest ways to publish an online service for an MVP (Minimum Viable Product). With pay-as-you-go pricing, you don’t have to worry about infrastructure and hosting. I’ll go into more detail, but these are definitely the best reasons that come to mind.
Azure Functions offers several key advantages:
- Ease of Use: Setting up and deploying an Azure Function is straightforward and quick. You can have a working endpoint in minutes, which is perfect for MVPs and prototypes.
- Cost Efficiency: Azure Functions can operate on a pay-as-you-go model. This means you only pay for the actual execution time of your functions, making it very cost-effective, especially for small projects or applications with variable traffic.
- No Infrastructure Management: With Azure Functions, you don’t need to worry about managing servers or infrastructure. Microsoft handles all the backend details, so you can focus entirely on writing code.
- Scalability: Azure Functions automatically scales to handle the load, whether you have a few requests or millions. This automatic scaling ensures that your application can handle growth seamlessly.
These features make Azure Functions an excellent choice for developers looking to quickly deploy and scale their applications without the hassle of managing infrastructure. Now, let’s dive into the specifics of how Azure Functions can make your development process smoother and more efficient.
What are Azure Functions?
Azure Functions is an on-demand cloud service that provides all the infrastructure and resources you need to run your applications, continuously updated and managed for you. It’s serverless platform as a service (PaaS), you can focus on writing the code that matters most to you, in your preferred programming language, while Azure Functions takes care of the rest. As a serverless compute service, Azure Functions allows you to build web APIs, respond to database changes, process IoT streams, manage message queues, and much more.
Azure Functions are Microsoft’s implementation of serverless functions; other alternatives include AWS Lambda, Google Cloud Functions, IBM Cloud Functions, Oracle Functions, Alibaba Cloud Function Compute, and Tencent Cloud SCF.
All these services allow you to use serverless functions in the cloud, but there are also implementations that work on-premises, such as OpenFaaS, Kubeless, Fission, and Knative.
What is platform as a service (PaaS)?
Platform as a Service (PaaS) is a cloud service that provides everything you need to develop and deploy applications. It includes resources like servers, storage, and networking, as well as tools for development, database management, and business intelligence. With PaaS, you pay for what you use and access everything over a secure Internet connection. It’s designed to support the entire lifecycle of web applications, from building and testing to deploying and managing.
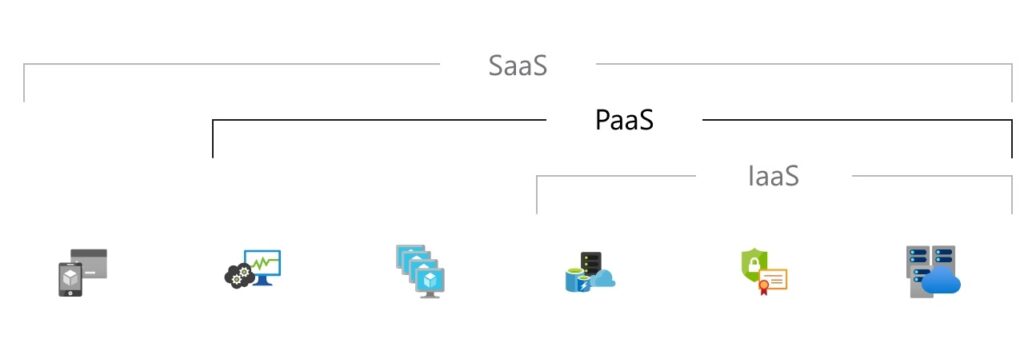
Scenarios for Using Azure Functions
Before we explore when to use Azure Functions, it’s important to know they’re not the solution for every problem. Understanding where they fit best is key. For me, I’ve used Azure Functions in different situations. I’ve quickly made scalable web APIs for my basic projects, set up scheduled tasks and got notifications when certain things happened in my database. Here are some scenarios where Azure Functions worked well for me:
Azure Functions Integration: Triggers and Bindings
In this section, we’ll explore one of the key strengths of Azure Functions: their seamless integration within the Azure ecosystem, facilitating effortless communication with various services like databases and more. The cornerstone of this integration lies in the concept of triggers and bindings. Triggers play a vital role in determining when a function is executed, acting as the catalyst for its invocation. Meanwhile, bindings serve as declarative connections to external resources, enabling data input and output operations without the need for hardcoded access.
Consider a scenario where you have a web application that requires real-time updates to a database whenever a new user registers. In this case, you can use an HTTP trigger to initiate the function whenever a new user submits their registration details. The function can then utilize a binding to seamlessly connect to the Azure Cosmos DB, enabling it to insert the new user’s data into the database without complex setup or configuration.
Another example could involve processing images uploaded to Azure Blob Storage. Here, a Blob Storage trigger can initiate the function whenever a new image is uploaded. By utilizing bindings, the function can then interact with Azure Cognitive Services to perform image analysis or with Azure SQL Database to store metadata associated with the uploaded images.
By leveraging triggers and bindings, Azure Functions provide a flexible and scalable solution for integrating with various Azure services and external systems. This not only simplifies the development process but also enhances the overall efficiency and reliability of your applications. As you explore Azure Functions further, understanding the intricacies of triggers and bindings will greatly influence the design and implementation of your functions, empowering you to create robust and seamlessly integrated solutions.
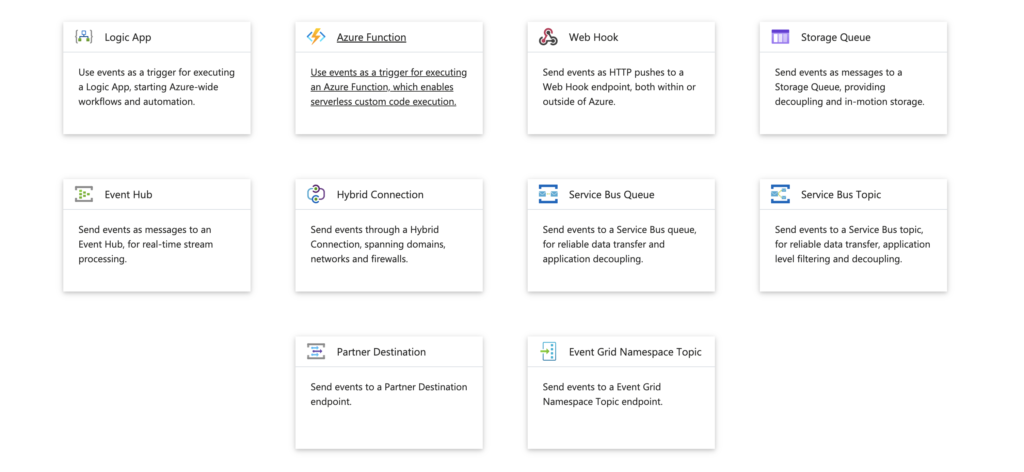
Azure Functions Hosting Options Overview
Understanding Azure Functions hosting options is essential as this flexibility comes with different costs. Azure Functions offer various hosting options, which you can explore in detail here. For personal projects, I typically choose the Consumption plan, while for work projects, I prefer the Premium plan due to its additional features. Here’s a brief overview of the hosting options:
- Consumption plan: Generally available (GA), no container support.
- Flex Consumption plan: Preview, no container support.
- Premium plan: Generally available (GA), supports Linux containers.
- Dedicated plan: Generally available (GA), supports Linux containers.
- Container Apps: Generally available (GA) through Azure Container Apps, supports Linux containers.
For more information, you can see here: https://learn.microsoft.com/en-us/azure/azure-functions/functions-scale#overview-of-plans
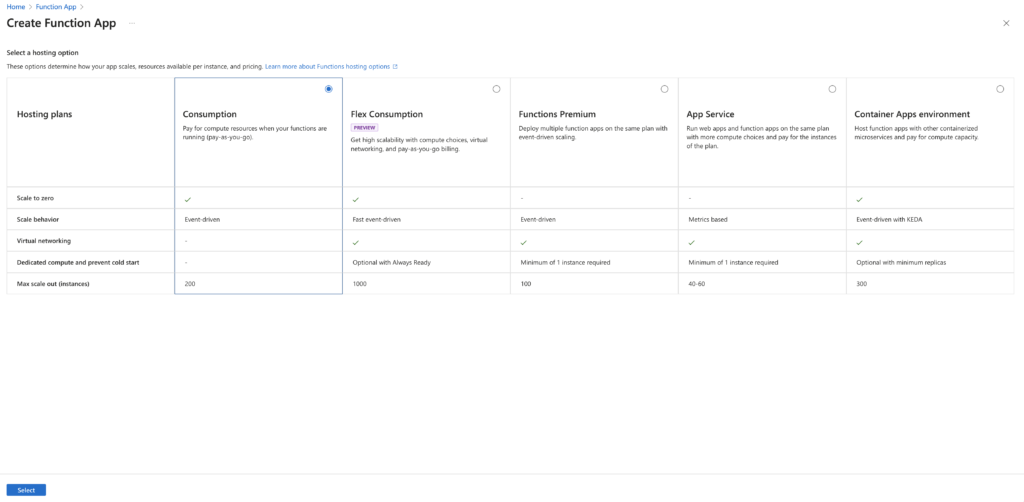
Creating a C# Function in Azure with Visual Studio Code
I hope you now understand why I love Azure Functions: it’s easy, flexible, and powerful. But to get the most out of it, you have to dive in. In this section, we’ll see how to create a C# function in Azure using Visual Studio Code. It’s a bit more hands-on compared to using Visual Studio, but don’t worry, I’ll walk you through it step by step.
Before you begin, ensure that you have the following prerequisites:
- Azure Account: You need an Azure account with an active subscription. If you don’t have one, you can create an account for free.
- .NET SDK: Make sure you have the .NET 8.0 SDK installed.
- Visual Studio Code: Install Visual Studio Code on your preferred platform.
- C# Extension: Install the C# extension for Visual Studio Code. This extension provides support for C# development in Visual Studio Code.
- Azure Functions Extension: Install the Azure Functions extension for Visual Studio Code. This extension enables you to create, debug, and deploy Azure Functions from within Visual Studio Code.
Here’s a brief overview of the steps:
Install or Update Core Tools
The Azure Functions extension for Visual Studio Code works with Azure Functions Core Tools, allowing you to run and debug your functions locally in Visual Studio Code using the Azure Functions runtime. Before you begin, it’s recommended to install or update Core Tools locally to ensure you have the latest version.
- Open Visual Studio Code and select F1 to open the command palette.
- Search for and run the command “Azure Functions: Install or Update Core Tools”.
- This command initiates the installation of the latest version of Core Tools.
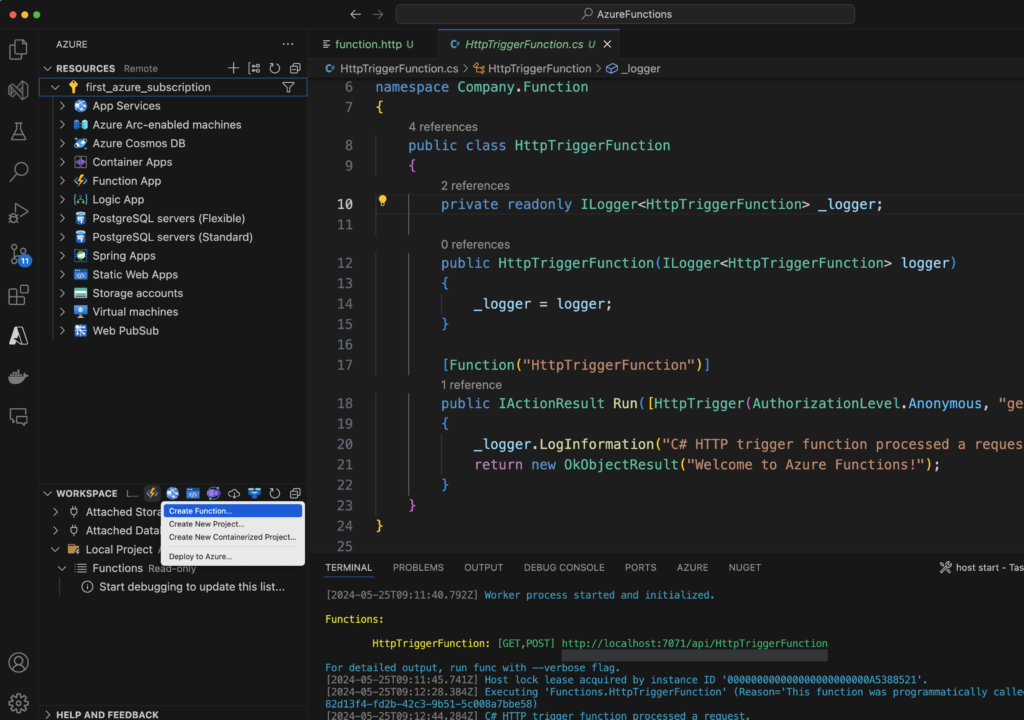
Create Your Local Project
In this section, you’ll use Visual Studio Code to create a local Azure Functions project in C#. Later, you’ll publish your function code to Azure.
- Click on the Azure icon in the Activity bar.
- In the Workspace (local) area, select the + button, then choose “Create Function” in the dropdown menu.
- When prompted, select “Create new project”.
- Choose the directory location for your project workspace and click “Select”. Ensure you either create a new folder or select an empty folder for the project workspace.
- Provide the following details at the prompts:
- Language: Choose “C#”.
- .NET Runtime: Choose “.NET 8.0 Isolated (LTS)”.
- Template: Choose “HTTP trigger”.
- Function Name: Type “HttpExample”.
- Namespace: Type “My.Functions”.
- Authorization Level: Choose “Anonymous” to enable anyone to call your function endpoint.
- Project Opening: Select “Open in current window”.
Run the Function Locally
Visual Studio Code integrates with Azure Functions Core Tools, allowing you to run your project on your local development computer before publishing it to Azure. If you haven’t already installed Core Tools locally, you’ll be prompted to install it the first time you run your project.
To execute your function, press F5 to start the function app project. The Terminal panel will display the output from Core Tools, and your app will start in the Terminal panel. You’ll be able to see the URL endpoint of your HTTP-triggered function running locally.
You can also create a .http
file to directly test the function call.
Final Thoughts
Looking back on my experience with Azure Functions, I can confidently say that I’m deeply impressed by their capabilities. I’ve been using them since version 1.0, despite their initial limitations. What I find most appealing is their versatility—they seamlessly run on both Windows and Linux environments, making them an ideal choice for rapid development without the hassle of setting up infrastructure.
Azure Functions have been my preferred solution for quickly launching projects, allowing me to bypass the complexities of traditional server setup. However, I recognize that certain economic factors and the push towards cloud adoption may hinder their availability for on-premises deployment. Nevertheless, there are plenty of alternatives available for those who require on-premises functionality.
In conclusion, Azure Functions have transformed the way I approach development tasks, offering unparalleled agility and scalability. While the dream of on-premises availability may remain elusive, the cloud-based flexibility and ease of use provided by Azure Functions continue to make them an indispensable tool in my development arsenal.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!