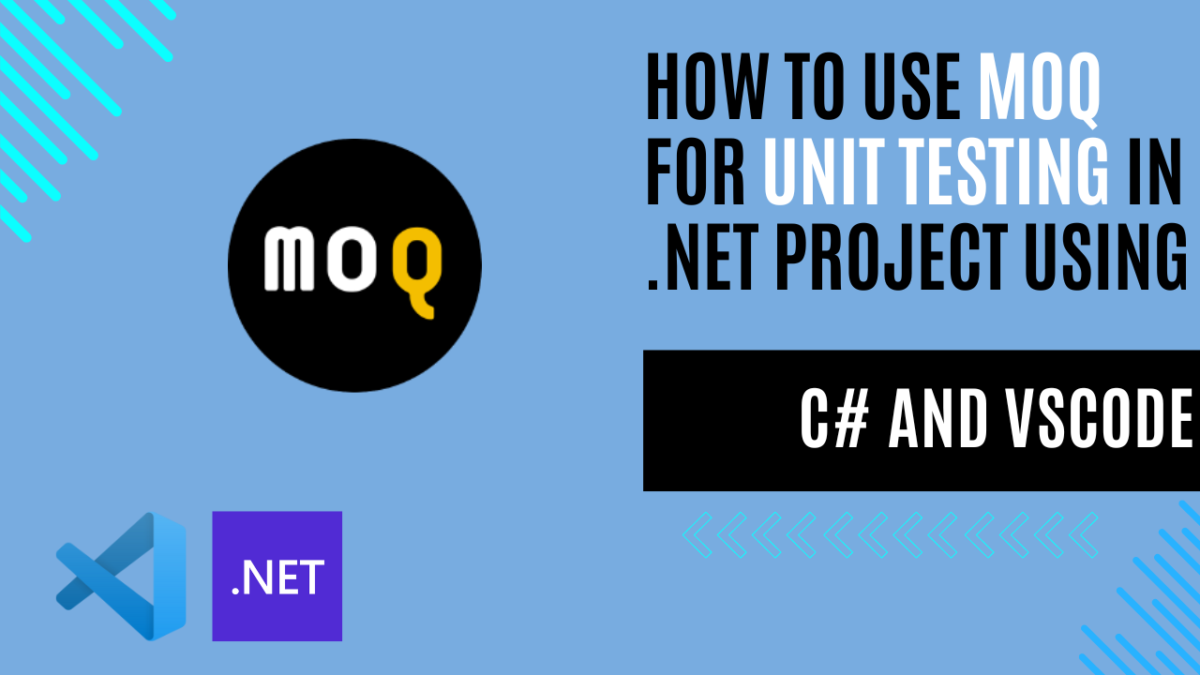
How to Use Moq for Unit Testing in .NET Projects Using C# in VSCode
Introduction
In the previous chapter, we discussed How to Use xUnit for Unit Testing in .NET Projects Using C# in VSCode, highlighting the importance of unit testing in software development. Today, we will dive into the Moq library, a powerful tool for creating mock objects in .NET unit tests. Moq allows developers to isolate and test specific components of their applications by simulating dependencies, making it easier to verify the behavior of individual units of code without relying on their external dependencies. This not only improves test accuracy but also enhances maintainability and robustness in software projects. Let’s explore how Moq can be integrated into your unit testing workflow to streamline testing and boost confidence in your codebase.
What is Moq and Why Use It?
What is Moq?
Moq (pronounced “Mock-you” or simply “Mock”) is a versatile and powerful mocking library specifically designed for .NET. Unlike other mocking frameworks, Moq was built from the ground up to fully leverage .NET’s Linq expression trees and lambda expressions. This design choice makes Moq exceptionally productive, type-safe, and refactoring-friendly. With Moq, developers can easily mock both interfaces and classes, making it a highly adaptable tool for a variety of testing scenarios. The library’s API is straightforward and intuitive, requiring little to no prior experience with mocking concepts, which lowers the entry barrier for new users.
Why Use Moq?
Moq was created to address the needs of developers who may not currently use a mocking library or find existing options too complex. It simplifies the process of creating mock objects, which are essential for isolating units of code during testing. By using Moq, developers can avoid the pitfalls of manual mocking, which often leads to cumbersome and less maintainable code. Moq’s design encourages a practical, unobtrusive approach to setting up dependencies in tests. Its API is designed to be user-friendly, allowing even novice developers to quickly become proficient and avoid common mistakes.
One of the standout features of Moq is its departure from the traditional Record/Replay approach used by other mocking libraries. Instead, Moq provides a more intuitive experience, making it easy to set up common expectations in a fixture setup method and override them as needed in specific unit tests. This flexibility not only improves test readability but also enhances the maintainability of test suites.
In summary, Moq is an essential tool for .NET developers seeking a simple, elegant, and efficient way to implement mocking in their unit tests. By leveraging the powerful features of C# and VB, Moq allows developers to create robust, type-safe tests that are easy to maintain and refactor.
o effectively use Moq, it is crucial to have a well-structured codebase that utilizes interfaces. This practice is particularly important when dealing with dependencies in software components.
Using Moq and the Importance of Interfaces
Dealing with Dependencies
In software development, most components rely on dependencies to perform their tasks. Delegating certain concerns to these dependencies not only clarifies the intent of each component but also allows developers to concentrate on their core responsibilities without being bogged down by implementation details. However, when writing unit tests, these dependencies need special attention to ensure that the tests focus solely on the behavior of the system under test (SUT) and do not inadvertently test the behavior of its dependencies.
The Role of Interfaces in Testability
One of the best practices for ensuring that a codebase is easily testable is adhering to the SOLID principles, particularly the Dependency Inversion Principle (DIP). According to DIP, high-level modules should not depend on low-level modules but should depend on abstractions, such as interfaces. By defining dependencies as interfaces, we can use Dependency Injection (DI) to provide these dependencies at runtime. This makes it easy to replace real implementations with mock objects during testing.
Mocks and Stubs
When a codebase is designed following these principles, developers can use two main techniques to handle dependencies in tests: stubs and mocks. Stubs are simplified versions of dependencies that mimic the behavior of the real objects, often used to provide controlled responses for specific test scenarios. Mocks, on the other hand, are more sophisticated and allow for the verification of interactions with the dependencies, such as checking whether certain methods were called with expected parameters.
Moq and the Power of Mocking
Moq excels in providing a streamlined and powerful way to create mocks for interface-based dependencies. It allows developers to focus on testing the SUT without worrying about the behavior of the actual dependencies. By leveraging Moq, you can easily set up expectations, configure return values, and verify interactions, ensuring that your tests are both isolated and precise. This not only improves test accuracy but also enhances the maintainability and flexibility of your code.
In conclusion, designing a codebase with a strong emphasis on interfaces and following best practices like SOLID principles is essential for making effective use of mocking frameworks like Moq. It simplifies the process of testing by allowing for the easy replacement of real dependencies with mocks, leading to more robust and reliable tests.
Example: How to Use Moq for Unit Testing
In the previous article, we discussed How to Use xUnit for Unit Testing, providing a foundational understanding of unit testing in .NET using xUnit. We shared a simple example of setting up a unit testing environment, emphasizing the importance of following best practices in coding and testing.
Disclaimer: This example is intended for educational purposes. While it provides a good starting point for learning, there are always better ways to write and optimize code and applications. Use this as a base and continually strive to follow best practices and improve your implementations.
GitHub Repository
All the code for this project is available in this GitHub repository. The repository includes the basic setup we previously created, and it’s structured to allow easy application of new modifications, utilizing tags and branches to navigate through different stages of the project.
In this example, we have a .NET solution with two projects: a console application called UnitTestConsoleApp
and a test project called UnitTestConsoleApp.Tests
. We use xUnit as our testing framework.
Prerequisites
Before starting, make sure you have the following installed:
- .NET SDK: Download and install the .NET SDK if you haven’t already.
- Visual Studio Code (VSCode): Install Visual Studio Code for a lightweight code editor.
- C# Extension for VSCode: Install the C# extension for VSCode to enable C# support.
Add the necessary NuGet packages for xUnit: dotnet add package Moq
Step 1: Update UnitTestSolution Example
Add IEmailService.cs
public interface IEmailService
{
bool SendEmail(string email, string subject, string body);
bool SendEmailWithAttachment(string email, string subject, string body, string attachmentPath);
}
Add EmailService.cs
public class EmailService : IEmailService
{
public bool SendEmail(string email, string subject, string body)
{
// Real implementation for sending an email
// For example, you might use an SMTP client
// Return true if the email is sent successfully, otherwise false
// Dummy logic for demonstration:
if (string.IsNullOrEmpty(email)) return false;
Console.WriteLine($"Sending email to {email} with subject '{subject}'");
return true; // Assume the email was sent successfully
}
public bool SendEmailWithAttachment(string email, string subject, string body, string attachmentPath)
{
// Logic for sending an email with attachments
// Dummy logic for demonstration:
if (string.IsNullOrEmpty(email) || string.IsNullOrEmpty(attachmentPath)) return false;
Console.WriteLine($"Sending email to {email} with attachment {attachmentPath}");
return true; // Assume the email with attachment was sent successfully
}
}
Implementing SendEmail and SendEmailWithAttachment Methods in the UserAccount Class
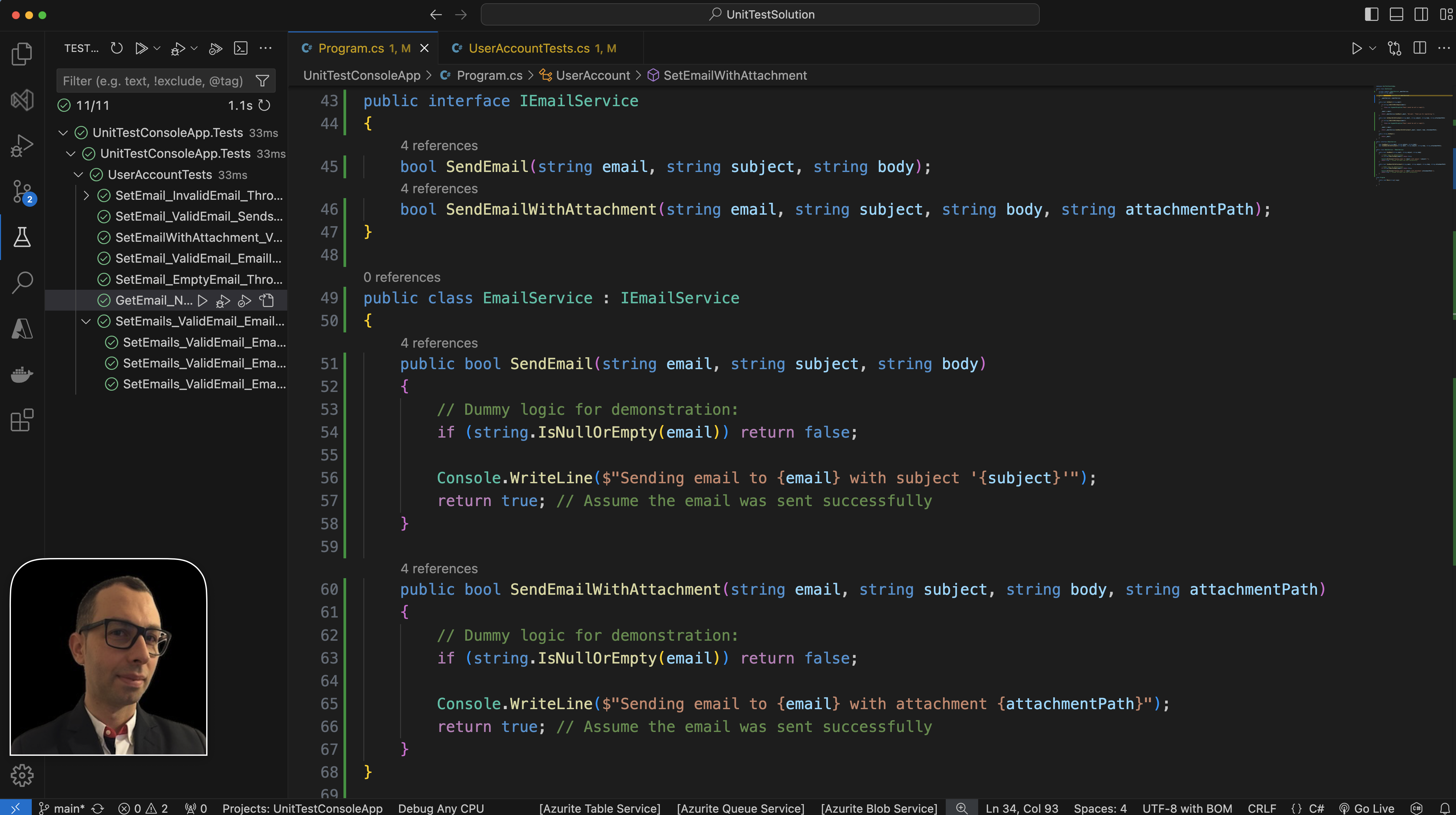
Here’s how the SendEmail
and SendEmailWithAttachment
methods should be implemented in the UserAccount
class to utilize the IEmailService
:
public class UserAccount
{
private string _email;
private readonly IEmailService _emailService;
public UserAccount(IEmailService emailService)
{
_emailService = emailService;
}
public bool SetEmail(string email)
{
if (string.IsNullOrWhiteSpace(email))
{
throw new ArgumentException("Email cannot be null or empty");
}
_email = email;
// Call the email service
return _emailService.SendEmail(_email, "Welcome", "Thank you for registering.");
}
public bool SetEmailWithAttachment(string email, string subject, string body, string attachmentPath)
{
if (string.IsNullOrWhiteSpace(email))
{
throw new ArgumentException("Email cannot be null or empty");
}
_email = email;
// Call the email service with an attachment
return _emailService.SendEmailWithAttachment(_email, subject, body, attachmentPath);
}
public string GetEmail()
{
return _email;
}
}
This implementation ensures that the UserAccount
class interacts with the IEmailService
to send emails and handle attachments, adhering to good design principles by allowing the email service to be easily mocked during testing.
Updated Test Class with Moq
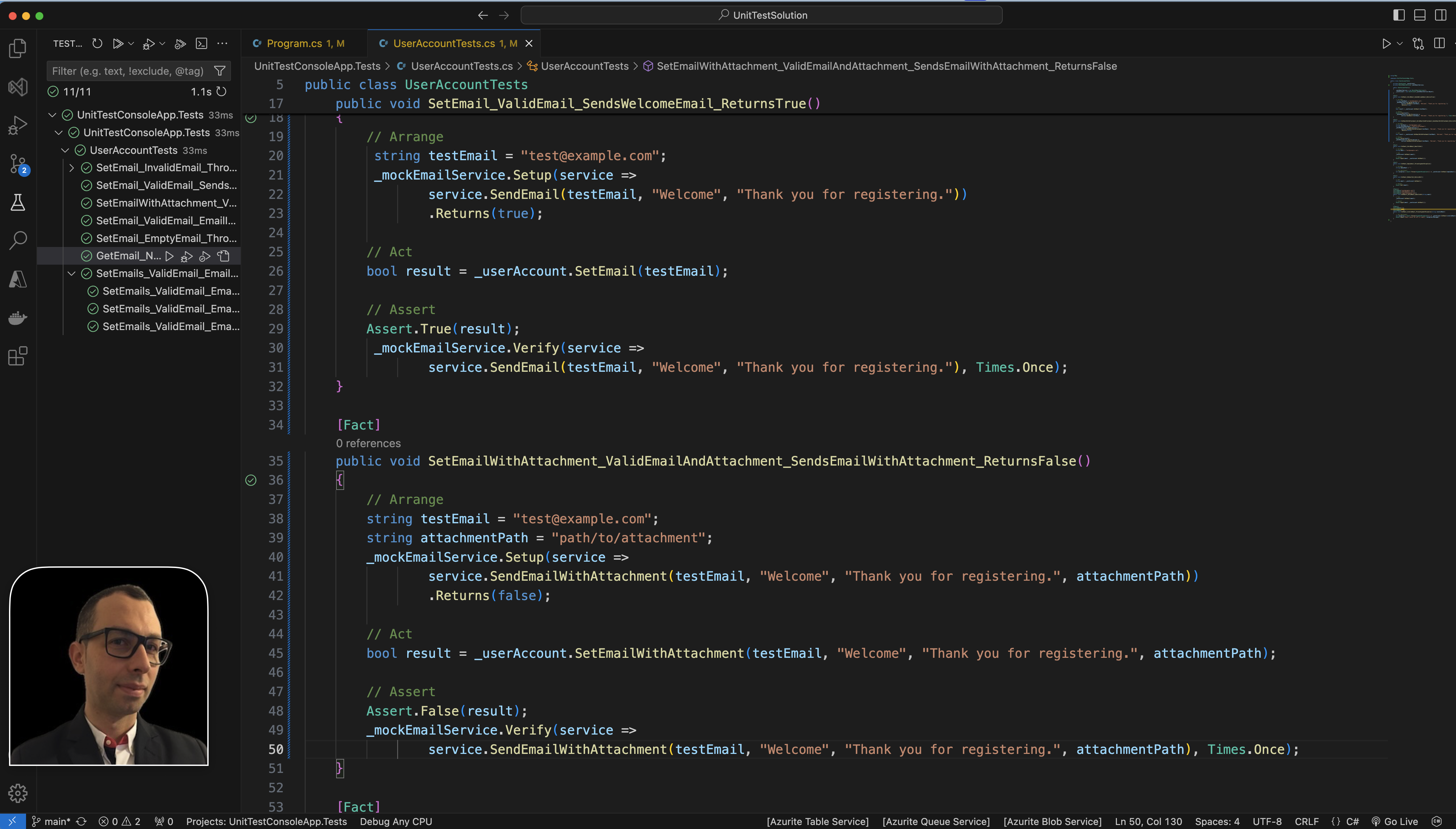
Here’s the updated UserAccountTests
class, reflecting the implementation of SendEmail
and SendEmailWithAttachment
methods:
using Moq;
namespace UnitTestConsoleApp.Tests;
public class UserAccountTests
{
private UserAccount _userAccount;
private Mock<IEmailService> _mockEmailService;
public UserAccountTests()
{
_mockEmailService = new Mock<IEmailService>();
_userAccount = new UserAccount(_mockEmailService.Object);
}
[Fact]
public void SetEmail_ValidEmail_SendsWelcomeEmail_ReturnsTrue()
{
// Arrange
string testEmail = "test@example.com";
_mockEmailService.Setup(service =>
service.SendEmail(testEmail, "Welcome", "Thank you for registering."))
.Returns(true);
// Act
bool result = _userAccount.SetEmail(testEmail);
// Assert
Assert.True(result);
_mockEmailService.Verify(service =>
service.SendEmail(testEmail, "Welcome", "Thank you for registering."), Times.Once);
}
[Fact]
public void SetEmailWithAttachment_ValidEmailAndAttachment_SendsEmailWithAttachment_ReturnsFalse()
{
// Arrange
string testEmail = "test@example.com";
string attachmentPath = "path/to/attachment";
_mockEmailService.Setup(service =>
service.SendEmailWithAttachment(testEmail, "Welcome", "Thank you for registering.", attachmentPath))
.Returns(false);
// Act
bool result = _userAccount.SetEmailWithAttachment(testEmail, "Welcome", "Thank you for registering.", attachmentPath);
// Assert
Assert.False(result);
_mockEmailService.Verify(service =>
service.SendEmailWithAttachment(testEmail, "Welcome", "Thank you for registering.", attachmentPath), Times.Once);
}
[Fact]
public void SetEmail_ValidEmail_EmailIsSet()
{
// Arrange
string email = "test@example.com";
// Act
_userAccount.SetEmail(email);
// Assert
Assert.Equal(email, _userAccount.GetEmail());
}
[Fact]
public void SetEmail_EmptyEmail_ThrowsArgumentException()
{
// Arrange
string emptyEmail = "";
// Act and Assert
var exception = Assert.Throws<ArgumentException>(() => _userAccount.SetEmail(emptyEmail));
}
[Fact]
public void GetEmail_NoEmailSet_ReturnsNull()
{
// Act
string email = _userAccount.GetEmail();
// Assert
Assert.Null(email);
}
[Theory]
[InlineData("user@example.com")]
[InlineData("admin@domain.com")]
[InlineData("support@company.org")]
public void SetEmails_ValidEmail_EmailIsSet(string email)
{
// Act
_userAccount.SetEmail(email);
// Assert
Assert.Equal(email, _userAccount.GetEmail());
}
[Theory]
[InlineData("")]
[InlineData(null)]
[InlineData(" ")]
public void SetEmail_InvalidEmail_ThrowsArgumentException(string invalidEmail)
{
// Act and Assert
var exception = Assert.Throws<ArgumentException>(() => _userAccount.SetEmail(invalidEmail));
Assert.Equal("Email cannot be null or empty", exception.Message);
}
}
Conclusion
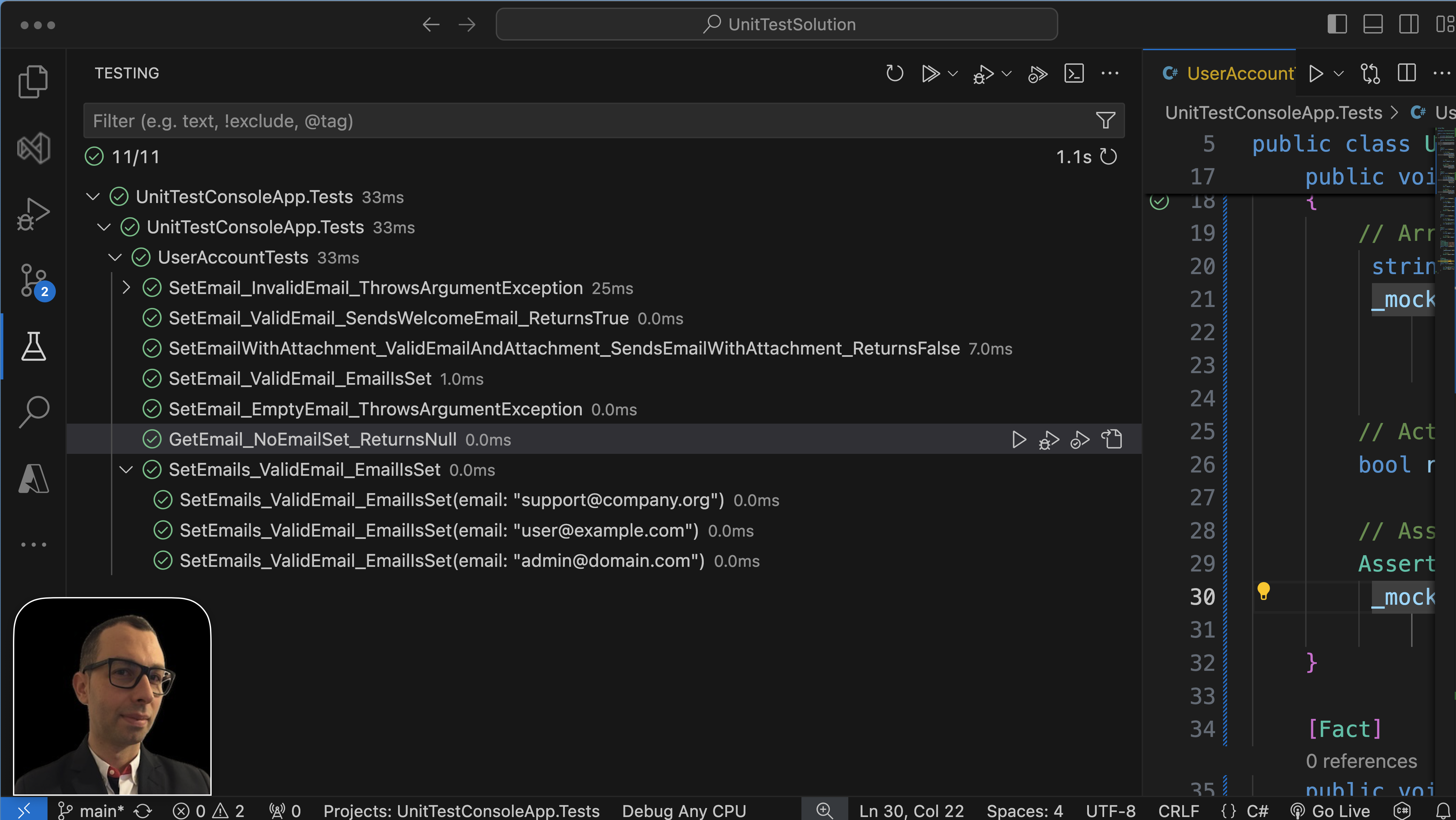
Writing unit tests is a cornerstone of effective software development, but it’s crucial to understand that having numerous tests alone does not ensure bug-free code. Unit tests play a significant role in identifying issues early and confirming that individual components perform as expected. Nonetheless, they represent just one facet of a well-rounded testing strategy.
Benefits of Unit Testing
- Early Detection of Bugs: Unit tests help detect errors in individual components before they have a chance to affect the entire application.
- Improved Code Quality: Writing tests promotes better design practices and encourages more modular code, which enhances readability and maintainability.
- Refactoring Confidence: With a comprehensive suite of unit tests, you can refactor your code confidently, knowing that any unintended changes will be caught by your tests.
- Documentation: Unit tests act as living documentation, showing how different parts of the application are supposed to function.
In this article, we have explored Moq, a powerful mocking library for .NET that simplifies the creation of mock objects in unit tests. Moq provides a straightforward way to simulate various scenarios and interactions between components, allowing you to write more thorough and flexible tests. By leveraging Moq, you can enhance your unit testing strategy, ensuring that your tests are both effective and maintainable.
Stay tuned for our next article, where we will delve deeper into advanced features and use cases of Moq, demonstrating how to fully utilize its capabilities to refine your testing practices.
If you think your friends or network would find this article useful, please consider sharing it with them. Your support is greatly appreciated.
Thanks for reading!